jinja2 tutorial
Python hosting: Host, run, and code Python in the cloud!
Jinja2 is a template engine for Python. You can use it when rendering data to web pages. For every link you visit, you want to show the data with the formatting. By using a template engine we can seperate display logic (html, css) from the actual Python code. Let’s start with an example
Related course
Python Flask: Make Web Apps with Python
Create the directories:
- /app
- /app/templates
And create the file user.html in /app/templates:
<title><block title %><endblock %></title> |
Then create the code app.py in /app/app.py:
from flask import Flask, flash, redirect, render_template, request |
Finally execute with:
python app.py |
You can then open http://127.0.0.1:8080/user/ in your browser. This will output the data formatted according to html:
<caption id=”attachment_1290” align=”alignnone” width=”637”]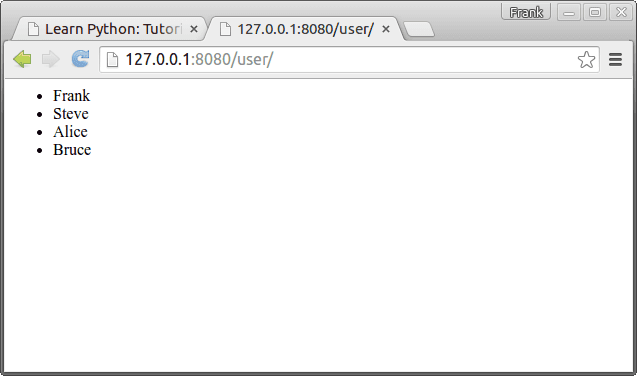
About Jinja
A Jinja2 template is simply a text file that does not need to have a specific extension such as .html, .xml.
A template may contain tags and special delimiters:
Delimiters | Usage |
---|---|
|
Statements |
|
Expressions to print to the template output |
|
Comments not included in the template output |
|
Line Statements |
In the example above we have two statements and one expression. We have not included any comments.
Base template and child templates
A Jinja2 template can extend a base template. On a webpage with many sites you may want these pages look similar. In /templates/ create a file called base.html with this code:
|
We did not set a style.css, but you could set one. Change /templates/user.html to:
<extends "base.html" %> |
Restart the app with:
python app.py |
Output:
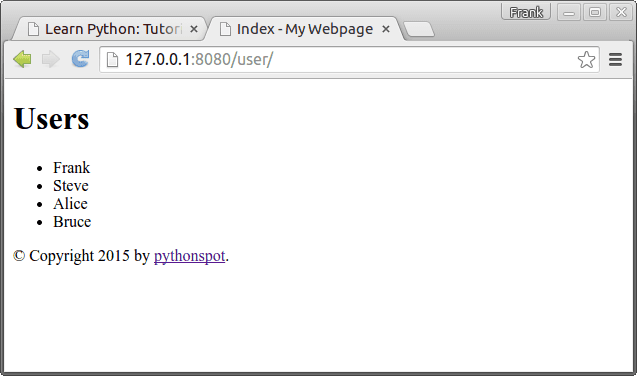
Leave a Reply:
When I used the template as suggested above, the browser[s] (Google Chrome 48.0.2564.116, and Firefox 38.5) translated the < and > correctly to ''. However, they failed to translate &;lt;ul> to (an un-ordered HTML list).
When I changed the template and replaced < with '' things clicked, and it worked as advertised.
I am using Python 3.5.1; Flask: Werkzeug/0.11.4
Thanks for your post! It should show the tags normally, I updated the source code.