pip install selenium
Python hosting: Host, run, and code Python in the cloud!
Selenium is a web automation tool. A web browser can be controlled using Python code, any task you would normally do on the web can be done using the selenium module.
To use use selenium, you need both the selenium module and the web driver installed. This can be quite tricky to get right, if you are new to selenium I recommend the course below.
Related course
Browser Automation with Python Selenium - Novice to Ninja
Install selenium
To get started, first you should setup a virtual environment. Once that’s setup and activated, you want to install the selenium module inside it. You can do that by typing the command:
|
This will install the selenium module, but that’s not all yet. You need to install the driver.
Then get the web driver from https://docs.seleniumhq.org/projects/webdriver. There are all kind of webdrivers including:
- ChromeDriver
- FirefoxDriver
- RemoteWebDriver
- EdgeDriver
- IEDriver
- SafariDriver
- OperaDriver
Selenium Example
Depending on which driver you install, you can load a different browser. If you use Chrome, you could do this:
from selenium.webdriver import Chrome |
For the Firefox driver, initialize like this:
from selenium.webdriver import Firefox |
This also works for Edge
from selenium.webdriver import Edge |
After installation of the web driver, we can make Python start the browser using the code below:
|
Save the program as example.py and run it with
python3 example.py |
If everything went right, it will start the Chromium browser and open the python site.
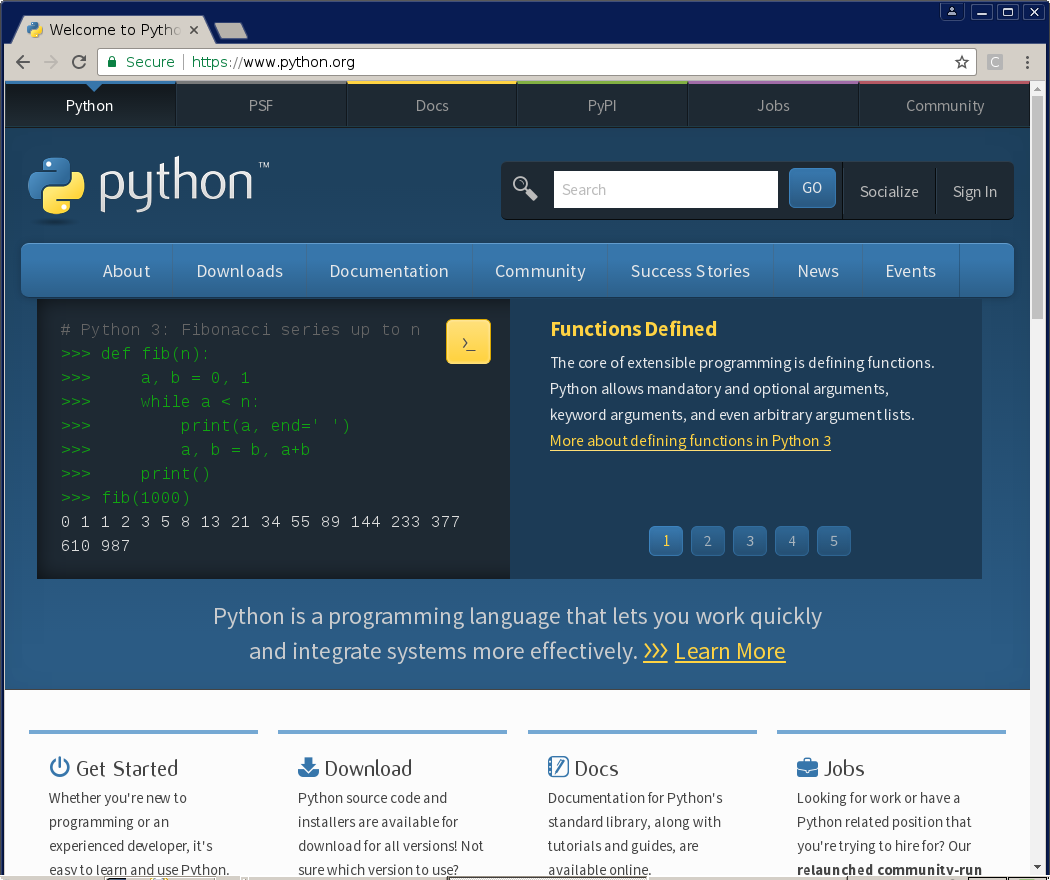
Leave a Reply: