pandas sqlite
Python hosting: Host, run, and code Python in the cloud!
An SQLite database can be read directly into Python Pandas (a data analysis library). In this article we’ll demonstrate loading data from an SQLite database table into a Python Pandas Data Frame. We’ll also briefly cover the creation of the sqlite database table using Python.
Related course
Data Analysis with Python Pandas
SQLite dataset
We create a simple dataset using this code:
|
It creates the SQLite database containing one table with dummy data.
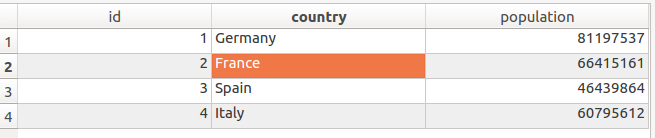
Sqlite to Python Panda Dataframe
An SQL query result can directly be stored in a panda dataframe:
|
We connect to the SQLite database using the line:
|
The line that converts SQLite data to a Panda data frame is:
|
where query is a traditional SQL query.
The dataframe (df) will contain the actual data.
Posted in pandas
Leave a Reply: