selenium click button
Python hosting: Host, run, and code Python in the cloud!
Selenium can automatically click on buttons that appear on a webpage. In this example we will open a site and click on a radio button and submit button.
Related course
Browser Automation with Python Selenium
Selenium button click
Start by importing the selenium module and creating a web driver object. We then use the method:
|
to find the html element. To get the path, we can use chrome development tools (press F12). We take the pointer in devtools and select the html button we are interested in. The path will then be shown, as the example screenshot:
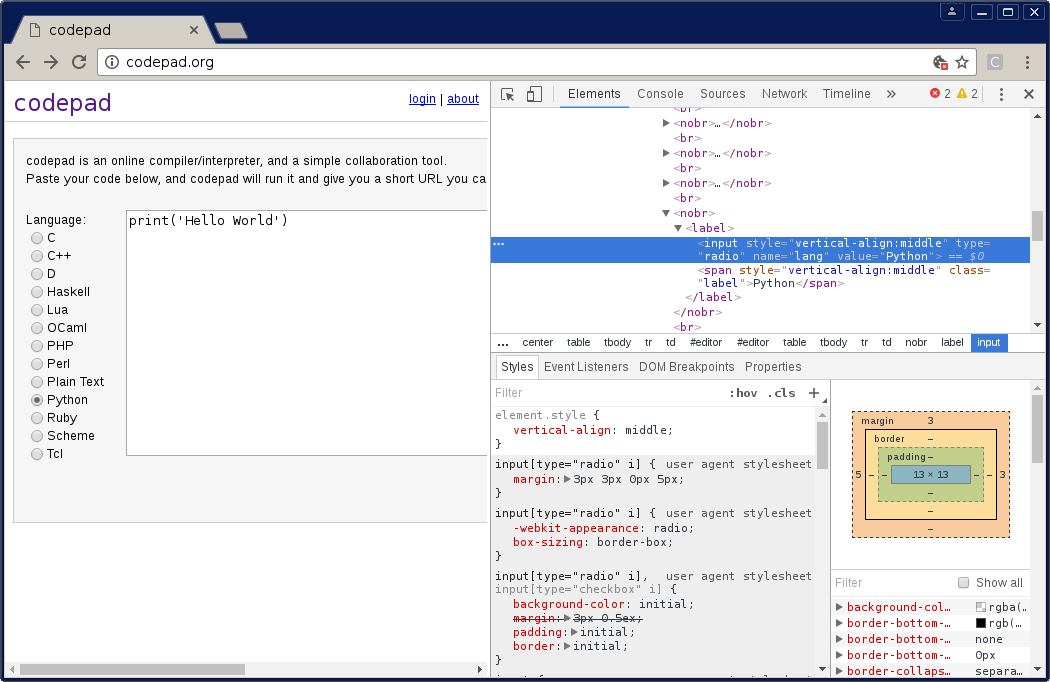
After we have the html object, we use the click() method to make the final click.
Full code:
|
Posted in selenium
Leave a Reply: