Tag: raw_input
Python hosting: Host, run, and code Python in the cloud!
Python variables
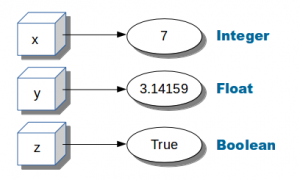
Variables can hold numbers that you can use one or more times.
Numbers can be of one of these datatypes:
- integer (1,2,3,4)
- float (numbers behind the dot)
- boolean (True or False)
Related Course:
Python Programming Bootcamp: Go from zero to hero
Numeric variables example
Example of numeric variables:
x = 1 y = 1.234 z = True |
You can output them to the screen using the print() function.
x = 1 y = 1.234 z = True print(x) print(y) print(z) |
Python supports arithmetic operations like addition (+), multiplication (*), division (/) and subtractions (-).
#!/usr/bin/env python x = 3 y = 8 sum = x + y print(sum) |
User input
Python 3
Use the input() function to get text input, convert to a number using int() or float().
#!/usr/bin/env python x = int(input("Enter x:")) y = int(input("Enter y:")) sum = x + y print(sum) |
Python 2 (old version)
You can also ask the user for input using the raw_input function:
#!/usr/bin/env python x = int(raw_input("Enter x:")) y = int(raw_input("Enter y:")) sum = x + y print(sum) |