Tag: web
pyqt5 webview
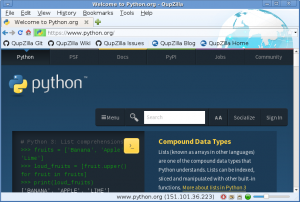
PyQt5 comes with a webkit webbrowser. Webkit is an open source web browser rendering engine that is used by Apple Safari and others. It was used in the older versions of Google Chrome, they have switched to the Blink rendering engine.
Related course:
Create GUI Apps with PyQt5
QWebView
The widget is called QWebView and webpages (HTML content) can be shown through this widget, local or live from the internet.
Methods
The QWebView class comes with a lot of methods including:
- back (self)
- forward (self)
- load (self, QUrl url)
- reload (self)
|
Related course:
Create GUI Apps with PyQt5
python web development
Flask with static html files
You can use the Flask framework and use static files together.
Flask will give you URL routing, many features and all the Python benefits.
You may want an application that is partly dynamic and partly static. Or you may simply want to browse with URL routing. In this article we will teach you how to load static HTML files with Flask.
Related course
Python Flask: Make Web Apps with Python
from flask import Flask, render_template |
This application initializes a Flask app with the method:
|
The app creates an URL route for any possible page and links that to static html files with:
|
</string:page_name>
Create a directory /templates/ and add the file hello.html:
|
Start the server with:
$ python app.py |
Then any .html file is accesible using URL routing.
For example the static file hello.html can be accessed using http://127.0.0.1:5000/hello. You can store any css file in the /static/ directory.