Category: beginner
Python hosting: Host, run, and code Python in the cloud!
Python dictionaries
A dictionary can be thought of as an unordered set of key: value pairs.
A pair of braces creates an empty dictionary: {}. Each element can maps to a certain value. An integer or string can be used for the index. Dictonaries do not have an order.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Dictionary example
Let us make a simple dictionary:
#!/usr/bin/python words = {} words["Hello"] = "Bonjour" words["Yes"] = "Oui" words["No"] = "Non" words["Bye"] = "Au Revoir" print(words["Hello"]) print(words["No"]) |
Output:
Bonjour Non |
We are by no means limited to single word defintions in the value part. A demonstration:
#!/usr/bin/python dict = {} dict['Ford'] = "Car" dict['Python'] = "The Python Programming Language" dict[2] = "This sentence is stored here." print(dict['Ford']) print(dict['Python']) print(dict[2]) |
Output:
Car
The Python Programming Language
This sentence is stored here. |
Manipulating the dictionary
We can manipulate the data stored in a dictionairy after declaration. This is shown in the example below:
#!/usr/bin/python words = {} words["Hello"] = "Bonjour" words["Yes"] = "Oui" words["No"] = "Non" words["Bye"] = "Au Revoir" print(words) # print key-pairs. del words["Yes"] # delete a key-pair. print(words) # print key-pairs. words["Yes"] = "Oui!" # add new key-pair. print(words) # print key-pairs. |
Output:
{'Yes': 'Oui', 'Bye': 'Au Revoir', 'Hello': 'Bonjour', 'No': 'Non'} {'Bye': 'Au Revoir', 'Hello': 'Bonjour', 'No': 'Non'} {'Yes': 'Oui!', 'Bye': 'Au Revoir', 'Hello': 'Bonjour', 'No': 'Non'} |
Datatype casting
Python determines the datatype automatically, to illustrate:
x = 3 y = "text" |
It finds x is of type integer and y of type string.
Functions accept a certain datatype. For example, print only accepts the string datatype.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Datatypes casting
If you want to print numbers you will often need casting.
In this example below we want to print two numbers, one whole number (integer) and one floating point number.
x = 3 y = 2.15315315313532 print("We have defined two numbers,") print("x = " + str(x)) print("y = " + str(y)) |
We cast the variable x (integer) and the variable y (float) to a string using the str() function.
What if we have text that we want to store as number? We will have to cast again.
a = "135.31421" b = "133.1112223" c = float(a) + float(b) print(c) |
In the example above we cast two variables with the datatype string to the datatype float.
Conversion functions
To convert between datatypes you can use:
Function | Description |
---|---|
int(x) | Converts x to an integer |
long(x) | Converts x to a long integer |
float(x) | Converts x to a floating point number |
str(x) | Converts x to an string. x can be of the type float. integer or long. |
hex(x) | Converts x integer to a hexadecimal string |
chr(x) | Converts x integer to a character |
ord(x) | Converts character x to an integer |
Random numbers
Using the random module, we can generate pseudo-random numbers. The function random() generates a random number between zero and one [0, 0.1 .. 1]. Numbers generated with this module are not truly random but they are enough random for most purposes.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Random number between 0 and 1.
We can generate a (pseudo) random floating point number with this small code:
from random import * print random() # Generate a pseudo-random number between 0 and 1. |
Generate a random number between 1 and 100
To generate a whole number (integer) between one and one hundred use:
from random import * print randint(1, 100) # Pick a random number between 1 and 100. |
This will print a random integer. If you want to store it in a variable you can use:
from random import * x = randint(1, 100) # Pick a random number between 1 and 100. print x |
Random number between 1 and 10
To generate a random floating point number between 1 and 10 you can use the uniform() function
from random import * print uniform(1, 10) |
Picking a random item from a list
Fun with lists
We can shuffle a list with this code:
from random import * items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] shuffle(items) print items |
To pick a random number from a list:
from random import * items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] x = sample(items, 1) # Pick a random item from the list print x[0] y = sample(items, 4) # Pick 4 random items from the list print y |
We can do the same thing with a list of strings:
from random import * items = ['Alissa','Alice','Marco','Melissa','Sandra','Steve'] x = sample(items, 1) # Pick a random item from the list print x[0] y = sample(items, 4) # Pick 4 random items from the list print y |
Read file
You have seen various types of data holders before: integers, strings, lists. But so far, we have not discussed how to read or write files.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Read file
You can read a file with the code below.
The file needs to be in the same directory as your program, if it is not you need to specify a path.
#!/usr/bin/env python # Define a filename. filename = "bestand.py" # Open the file as f. # The function readlines() reads the file. with open(filename) as f: content = f.readlines() # Show the file contents line by line. # We added the comma to print single newlines and not double newlines. # This is because the lines contain the newline character '\n'. for line in content: print(line), |
The first part of the code will read the file content. All of the lines read will be stored in the variable content. The second part will iterate over every line in the variable contents.
If you do not want to read the newline characters ‘\n’, you can change the statement f.readlines() to this:
content = f.read().splitlines() |
Resulting in this code:
#!/usr/bin/env python # Define a filename. filename = "bestand.py" # Open the file as f. # The function readlines() reads the file. with open(filename) as f: content = f.read().splitlines() # Show the file contents line by line. # We added the comma to print single newlines and not double newlines. # This is because the lines contain the newline character '\n'. for line in content: print(line) |
While the codes above work, we should always test if the file we want to open exists. We will test first if the file does not exist, if it does it will read the file else return an error. As in the code below:
#!/usr/bin/env python import os.path # Define a filename. filename = "bestand.py" if not os.path.isfile(filename): print 'File does not exist.' else: # Open the file as f. # The function readlines() reads the file. with open(filename) as f: content = f.read().splitlines() # Show the file contents line by line. # We added the comma to print single newlines and not double newlines. # This is because the lines contain the newline character '\n'. for line in content: print(line) |
Write file
Python supports writing files by default, no special modules are required. You can write a file using the .write() method with a parameter containing text data.
Before writing data to a file, call the open(filename,’w’) function where filename contains either the filename or the path to the filename. Finally, don’t forget to close the file.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Create file for writing
The code below creates a new file (or overwrites) with the data.
#!/usr/bin/env python # Filename to write filename = "newfile.txt" # Open the file with writing permission myfile = open(filename, 'w') # Write a line to the file myfile.write('Written with Python\n') # Close the file myfile.close() |
The ‘w’ flag makes Python truncate the file if it already exists. That is to say, if the file contents exists it will be replaced.
Append to file
If you simply want to add content to the file you can use the ‘a’ parameter.
#!/usr/bin/env python # Filename to append filename = "newfile.txt" # The 'a' flag tells Python to keep the file contents # and append (add line) at the end of the file. myfile = open(filename, 'a') # Add the line myfile.write('Written with Python\n') # Close the file myfile.close() |
Parameters
A summary of parameters:
Flag | Action |
---|---|
r | Open file for reading |
w | Open file for writing (will truncate file) |
b | binary more |
r+ | open file for reading and writing |
a+ | open file for reading and writing (appends to end) |
w+ | open file for reading and writing (truncates files) |
Python class: Objects and classes
Introduction
Technology always evolves. What are classes and where do they come from?
1. Statements:
In the very early days of computing, programmers wrote only commands.
2. Functions:
Reusable group of statements, helped to structure that code and it improved readability.
3. Classes:
Classes are used to create objects which have functions and variables. Strings are examples of objects: A string book has the functions book.replace() and book.lowercase(). This style is often called object oriented programming.
Lets take a dive!
Python Courses:
Python Programming Bootcamp: Go from zero to hero
Python class
We can create virtual objects in Python. A virtual object can contain variables and methods. A program may have many different types and are created from a class. Example:
class User: name = "" def __init__(self, name): self.name = name def sayHello(self): print "Hello, my name is " + self.name # create virtual objects james = User("James") david = User("David") eric = User("Eric") # call methods owned by virtual objects james.sayHello() david.sayHello() |
Run this program. In this code we have 3 virtual objects: james, david and eric. Each object is instance of the User class.
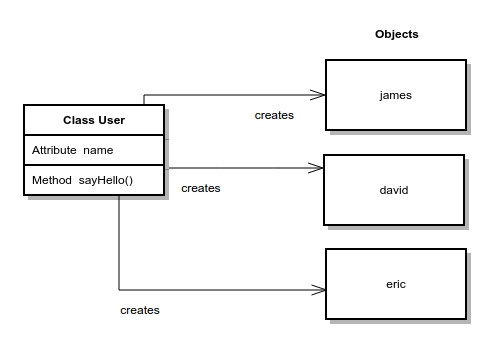
In this class we defined the sayHello() method, which is why we can call it for each of the objects. The __init__() method is called the constructor and is always called when creating an object. The variables owned by the class is in this case “name”. These variables are sometimes called class attributes.
We can create methods in classes which update the internal variables of the object. This may sound vague but I will demonstrate with an example.
Class variables
We define a class CoffeeMachine of which the virtual objects hold the amount of beans and amount of water. Both are defined as a number (integer). We may then define methods that add or remove beans.
def addBean(self): self.bean = self.bean + 1 def removeBean(self): self.bean = self.bean - 1 |
We do the same for the variable water. As shown below:
class CoffeeMachine: name = "" beans = 0 water = 0 def __init__(self, name, beans, water): self.name = name self.beans = beans self.water = water def addBean(self): self.beans = self.beans + 1 def removeBean(self): self.beans = self.beans - 1 def addWater(self): self.water = self.water + 1 def removeWater(self): self.water = self.water - 1 def printState(self): print "Name = " + self.name print "Beans = " + str(self.beans) print "Water = " + str(self.water) pythonBean = CoffeeMachine("Python Bean", 83, 20) pythonBean.printState() print "" pythonBean.addBean() pythonBean.printState() |
Run this program. The top of the code defines the class as we described. The code below is where we create virtual objects. In this example we have exactly one object called “pythonBean”. We then call methods which change the internal variables, this is possible because we defined those methods inside the class. Output:
Name = Python Bean Beans = 83 Water = 20 Name = Python Bean Beans = 84 Water = 20 |