Category: beginner
Python hosting: Host, run, and code Python in the cloud!
Python set
Sets in Python
A set in Python is a collection of objects. Sets are available in Python 2.4 and newer versions. They are different from lists or tuples in that they are modeled after sets in mathematics.
Related course
Python Programming Bootcamp: Go from zero to hero
Set example
To create a set, we use the set() function.
#!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram"]) print(x) |
If we add the same item element multiple times, they are removed. A set may not contain the same element multiple times.
#!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram", "Postcard"]) print(x) |
Related Courses:
Simple notation
If you use Python version 2.6 or a later version, you can use a simplified notation:
#!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram"]) print(x) y = {"Postcard","Radio","Telegram"} print(y) |
Set Methods
Clear elements from set
To remove all elements from sets:
#!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram"]) x.clear() print(x) |
Add elements to a set
To add elements to a set:
#!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram"]) x.add("Telephone") print(x) |
Remove elements to a set
To remove elements to a set:
!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram"]) x.remove("Radio") print(x) |
Difference between two sets
To find the difference between two sets use:
#!/usr/bin/env python x = set(["Postcard", "Radio", "Telegram"]) y = set(["Radio","Television"]) print( x.difference(y) ) print( y.difference(x) ) |
Be aware that x.difference(y) is different from y.difference(x).
Subset
To test if a set is a subset use:
#!/usr/bin/env python x = set(["a","b","c","d"]) y = set(["c","d"]) print( x.issubset(y) )<b> </b> |
Super-set
To test if a set is a super-set:
#!/usr/bin/env python x = set(["a","b","c","d"]) y = set(["c","d"]) print( x.issuperset(y) ) |
Intersection
To test for intersection, use:
#!/usr/bin/env python x = set(["a","b","c","d"]) y = set(["c","d"]) print( x.intersection(y) ) |
Python modules
Modular programming
As you are programming, the software can quickly scale into a large code base. To manage complexity we can use classes, functions and modules.
Related course
Python Programming Bootcamp: Go from zero to hero
Module content
To show the accessible functions (and included variables) in a module, you can use this code:
#!/usr/bin/env python import sys print(dir(sys)) |
Result:
['__displayhook__', '__doc__', '__egginsert', '__excepthook__', '__name__', '__package__', '__plen', '__stderr__', '__stdin__', '__stdout__', '_clear_type_cache', '_current_frames', '_getframe', '_mercurial', '_multiarch', 'api_version', 'argv', 'builtin_module_names', 'byteorder', 'call_tracing', 'callstats', 'copyright', 'displayhook', 'dont_write_bytecode', 'exc_clear', 'exc_info', 'exc_type', 'excepthook', 'exec_prefix', 'executable', 'exit', 'flags', 'float_info', 'float_repr_style' , 'getcheckinterval', 'getdefaultencoding', 'getdlopenflags', 'getfilesystemencoding', 'getprofile', 'getrecursionlimit', 'getrefcount', 'getsizeof', 'gettrace', 'hexversion', 'long_info', 'maxint', 'maxsize', 'maxunicode', 'meta_path', 'modules', 'path', 'path_hooks', 'path_importer_cache', 'platform', 'prefix', 'py3kwarning', 'pydebug', 'setcheckinterval', 'setdlopenflags' , 'setprofile', 'setrecursionlimit', 'settrace', 'stderr', 'stdin', 'stdout', 'subversion', 'version', 'version_info', 'warnoptions'] |
Create a Module
You can create your own module in these steps:
Create a file called test.py (your module)
#!/usr/bin/env python def add(a,b): return a+b |
Then create a file called app.py:
from test import * print('hello') print(add(5,2)) |
Python graph
Introduction
A graph in mathematics and computer science consists of “nodes” which may or may not be connected with one another. Connections between nodes are called edges. A graph can be directed (arrows) or undirected. The edges could represent distance or weight.
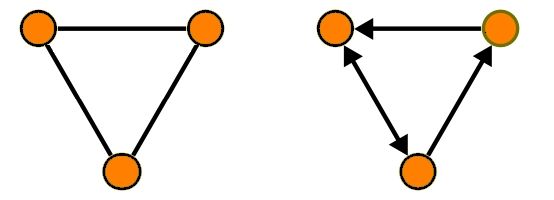
default graph (left), directed graph (right)
Python does not have a graph data type. To use graphs we can either use a module or implement it ourselves:
- implement graphs ourselves
- networkx module
Related course
Python Programming Bootcamp: Go from zero to hero
Graph in Python
A directed graph can be defined as:
#!/usr/bin/env python graph = {'A': ['B', 'C'], 'B': ['C', 'A'], 'C': ['D'], 'D': ['A']} print(graph) |
Graphs using networkx
The networkx software module has support for creating, manipulating graphs.
#!/usr/bin/env python import networkx as nx G=nx.Graph() G.add_node("A") G.add_node("B") G.add_node("C") G.add_edge("A","B") G.add_edge("B","C") G.add_edge("C","A") print("Nodes: " + str(G.nodes())) print("Edges: " + str(G.edges())) |
Result:
Nodes: ['A', 'C', 'B'] Edges: [('A', 'C'), ('A', 'B'), ('C', 'B')]
Python finite state machine
Introduction
A finite state machine (FSM) is a mathematical model of computation with states, transitions, inputs and outputs. This machine is always in a one state at the time and can move to other states using transitions. A transition changes the state of the machine to another state.
A large number of problems can be modeled using finite state machines. Simple examples of state machines used in modern life are vending machines, elevators and traffic lights. Advanced usage are artificial intelligence, language parsing and communication protocol design.
Related course
Python Programming Bootcamp: Go from zero to hero
Finite State Machine Example
First install the Fysom module:
sudo pip install fysom
We can define a Finite State Machine (FSM) with two states: sleeping and awake. To move between the states we will define the transitions wakeup() and sleep().
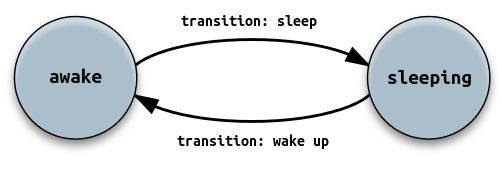
Example:
from fysom import * fsm = Fysom({'initial': 'awake', 'final': 'red', 'events': [ {'name': 'wakeup', 'src': 'sleeping', 'dst': 'awake'}, {'name': 'sleep', 'src': 'awake', 'dst': 'sleeping'}]}) print(fsm.current) # awake fsm.sleep() print(fsm.current) # sleeping fsm.wakeup() print(fsm.current) # awake |
Result:
awake sleeping awake |
Finite State Machines
There are several implementations of Finite State Machines in Python:
Python tree
Introduction
In computer science, a tree is a data structure that is modeled after nature. Unlike trees in nature, the tree data structure is upside down: the root of the tree is on top. A tree consists of nodes and its connections are called edges. The bottom nodes are also named leaf nodes. A tree may not have a cycle.
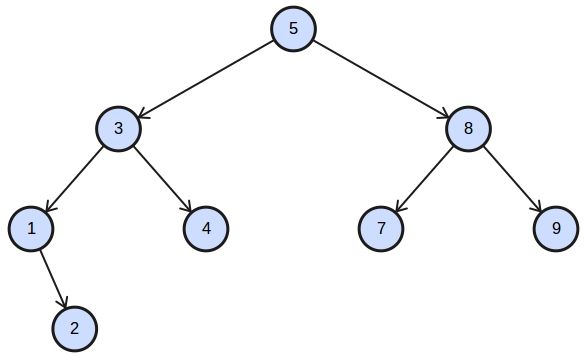
Python does not have built-in support for trees.
Related Courses:
Binary tree
A binary tree is a data structure where every node has at most two children (left and right child). The root of a tree is on top. Every node below has a node above known as the parent node.We define a class thee which has a left and right attribute. From this binary tree we define the root (top of the three) and a left and right node.
#!/usr/bin/env python class Tree(object): def __init__(self): self.left = None self.right = None self.data = None root = Tree() root.data = "root" root.left = Tree() root.left.data = "left" root.right = Tree() root.right.data = "right" print(root.left.data) |
You could then further create the tree like this:
#!/usr/bin/env python class Tree(object): def __init__(self): self.left = None self.right = None self.data = None root = Tree() root.data = "root" root.left = Tree() root.left.data = "left" root.right = Tree() root.right.data = "right" root.left.left = Tree() root.left.left.data = "left 2" root.left.right = Tree() root.left.right.data = "left-right" |
Binary numbers and logical operators
More detailed: While this is not directly useful in web applications or most desktop applications, it is very useful to know.
In this article you will learn how to use binary numbers in Python, how to convert them to decimals and how to do bitwise operations on them.
Related course:
Python Programming Bootcamp: Go from zero to hero
Binary numbers
At the lowest level, the computer has no notion whatsoever of numbers except ‘there is a signal’ or ‘these is not a signal’. You can think of this as a light switch: Either the switch is on or it is off.
This tiny amount of information, the smallest amount of information that you can store in a computer, is known as a bit. We represent a bit as either low (0) or high (1).
To represent higher numbers than 1, the idea was born to use a sequence of bits. A sequence of eight bits could store much larger numbers, this is called a byte. A sequence consisting of ones and zeroes is known as binary. Our traditional counting system with ten digits is known as decimal.
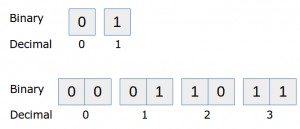
Lets see that in practice:
# Prints out a few binary numbers. print int('00', 2) print int('01', 2) print int('10', 2) print int('11', 2) |
The second parameter 2, tells Python we have a number based on 2 elements (1 and 0). To convert a byte (8 bits) to decimal, simple write a combination of eight bits in the first parameter.
# Prints out a few binary numbers. print int('00000010', 2) # outputs 2 print int('00000011', 2) # outputs 3 print int('00010001', 2) # outputs 17 print int('11111111', 2) # outputs 255 |
How does the computer do this? Every digit (from right to left) is multiplied by the power of two.
The number ‘00010001‘ is (1 x 2^0) + (0 x 2^1) + (0 x 2^2) + (0 x 2^3) + (1 x 2^4) + (0 x 2^5) + (0 x 2^6) + (0 x 2^7) = 16 + 1 = 17. Remember, read from right to left.
The number ‘00110010’ would be (0 x 2^0) + (1 x 2^1) + (0 x 2^2) + (0 x 2^3) + (1 x 2^4) + (1 x 2^5) + (0 x 2^6) + (0 x 2^7) = 32+16+2 = 50.
Try the sequence ‘00101010’ yourself to see if you understand and verify with a Python program.
Logical operations with binary numbers
Binary Left Shift and Binary Right Shift
Multiplication by a factor two and division by a factor of two is very easy in binary. We simply shift the bits left or right. We shift left below:
Bit 4 | Bit 3 | Bit 2 | Bit 1 |
---|---|---|---|
0 | 1 | 0 | 1 |
1 | 0 | 1 | 0 |
Before shifting (0,1,0,1) we have the number 5 . After shifting (1,0,1,0) we have the number 10. In python you can use the bitwise left operator (&l;<) to shift left and the bitwise right operator (>>) to shift right.
inputA = int('0101',2) print "Before shifting " + str(inputA) + " " + bin(inputA) print "After shifting in binary: " + bin(inputA << 1) print "After shifting in decimal: " + str(inputA << 1) |
Output:
Before shifting 5 0b101 After shifting in binary: 0b1010 After shifting in decimal: 10 |
The AND operator
Given two inputs, the computer can do several logic operations with those bits. Let’s take the AND operator. If input A and input B are positive, the output will be positive. We will demonstrate the AND operator graphically, the two left ones are input A and input B, the right circle is the output:
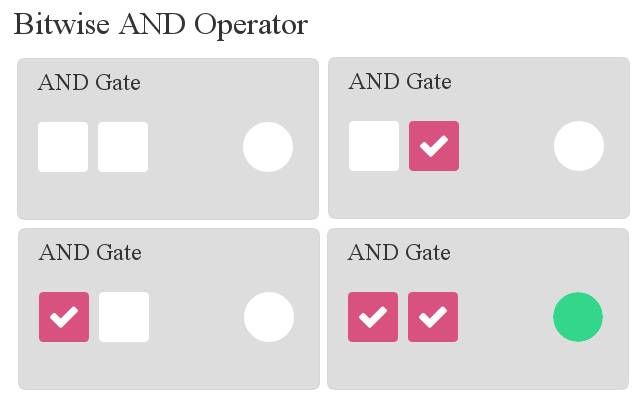
In code this is as simple as using the & symbol, which represents the Logical AND operator.
# This code will execute a bitwise logical AND. Both inputA and inputB are bits. inputA = 1 inputB = 1 print inputA & inputB # Bitwise AND |
By changing the inputs you will have the same results as the image above. We can do the AND operator on a sequence:
inputA = int('00100011',2) # define binary sequence inputA inputB = int('00101101',2) # define binary sequence inputB print bin(inputA & inputB) # logical AND on inputA and inputB and output in binary |
Output:
0b100001 # equals 00100001 |
This makes sense because if you do the operation by hand:
00100011 00101101 -------- Logical bitwise AND 00100001 |
The OR operator
Now that you have learned the AND operator, let’s have a look at the OR operator. Given two inputs, the output will be zero only if A and B are both zero.
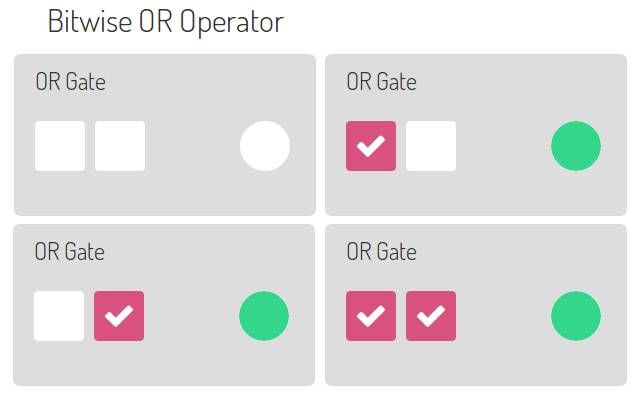
To execute it, we use the | operator. A sequence of bits can simply be executed like this:
inputA = int('00100011',2) # define binary number inputB = int('00101101',2) # define binary number print bin(inputA) # prints inputA in binary print bin(inputB) # prints inputB in binary print bin(inputA | inputB) # Execute bitwise logical OR and print result in binary |
Output:
0b100011 0b101101 0b101111 |
The XOR operator
This is an interesting operator: The Exclusive OR or shortly XOR.
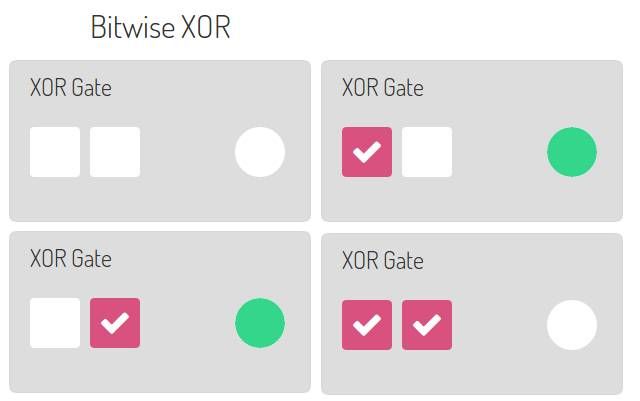
To execute it, we use the ^ operator. A sequence of bits can simply be executed like this:
inputA = int('00100011',2) # define binary number inputB = int('00101101',2) # define binary number print bin(inputA) # prints inputA in binary print bin(inputB) # prints inputB in binary print bin(inputA ^ inputB) # Execute bitwise logical OR and print result in binary |
Output:
0b100011 0b101101 0b1110 |