Category: beginner
Python hosting: Host, run, and code Python in the cloud!
Functions
A function is reusable code that can be called anywhere in your program. Functions improve readability of your code: it’s easier for someone to understand code using functions instead of long lists of instructions.
On top of that, functions can be reused or modified which also improve testability and extensibility.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Function definition
We use this syntax to define as function:
def function(parameters): instructions return value |
The def keyword tells Python we have a piece of reusable code (A function). A program can have many functions.
Practical Example
We can call the function using function(parameters).
#!/usr/bin/python def f(x): return(x*x) print(f(3)) |
Output:
9 |
The function has one parameter, x. The return value is the value the function returns. Not all functions have to return something.
Parameters
We can pass multiple variables:
#!/usr/bin/python def f(x,y): print('You called f(x,y) with the value x = ' + str(x) + ' and y = ' + str(y)) print('x * y = ' + str(x*y)) f(3,2) |
Output:
You called f(x,y) with the value x = 3 and y = 2 x * y = 6 |
Global and Local variables
There are two types of variables: global variables and local variables.
A global variable can be reached anywhere in the code, a local only in the scope.
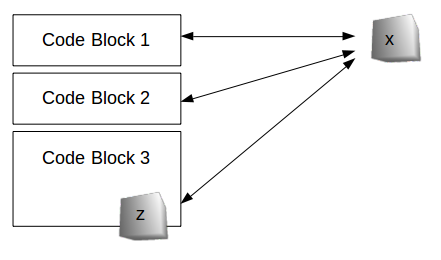
Related Course:
Python Programming Bootcamp: Go from zero to hero
Local variables
Local variables can only be reached in their scope.
The example below has two local variables: x and y.
def sum(x,y): sum = x + y return sum print(sum(8,6)) |
The variables x and y can only be used inside the function sum, they don’t exist outside of the function.
Local variables cannot be used outside of their scope, this line will not work:
print(x) |
Global variables
A global variable can be used anywhere in the code.
In the example below we define a global variable z
z = 10 def afunction(): global z print(z) afunction() print(z) |
The global variable z can be used all throughout the program, inside functions or outside.
A global variable can modified inside a function and change for the entire program:
z = 10 def afunction(): global z z = 9 afunction() print(z) |
After calling afunction(), the global variable is changed for the entire program.
Exercise
Local and global variables can be used together in the same program.
Try to determine the output of this program:
z = 10 def func1(): global z z = 3 def func2(x,y): global z return x+y+z func1() total = func2(4,5) print(total) |
Scope
Scope
Variables can only reach the area in which they are defined, which is called scope. Think of it as the area of code where variables can be used. Python supports global variables (usable in the entire program) and local variables.
By default, all variables declared in a function are local variables. To access a global variable inside a function, it’s required to explicitly define ‘global variable’.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Example
Below we’ll examine the use of local variables and scope. This will not work:
#!/usr/bin/python def f(x,y): print('You called f(x,y) with the value x = ' + str(x) + ' and y = ' + str(y)) print('x * y = ' + str(x*y)) z = 4 # cannot reach z, so THIS WON'T WORK z = 3 f(3,2) |
but this will:
#!/usr/bin/python def f(x,y): z = 3 print('You called f(x,y) with the value x = ' + str(x) + ' and y = ' + str(y)) print('x * y = ' + str(x*y)) print(z) # can reach because variable z is defined in the function f(3,2) |
Let’s examine this further:
#!/usr/bin/python def f(x,y,z): return x+y+z # this will return the sum because all variables are passed as parameters sum = f(3,2,1) print(sum) |
Calling functions in functions
We can also get the contents of a variable from another function:
#!/usr/bin/python def highFive(): return 5 def f(x,y): z = highFive() # we get the variable contents from highFive() return x+y+z # returns x+y+z. z is reachable becaue it is defined above result = f(3,2) print(result) |
If a variable can be reached anywhere in the code is called a global variable. If a variable is known only inside the scope, we call it a local variable.
Loops: For loop, while loop
Code can be repeated using a loop. Lines of code can be repeated N times, where N is manually configurable. In practice, it means code will be repeated until a condition is met. This condition is usually (x >=N) but it’s not the only possible condition.
Python has 3 types of loops: for loops, while loops and nested loops.
Related Course:
Python Programming Bootcamp: Go from zero to hero
For loop
We can iterate a list using a for loop
#!/usr/bin/python items = [ "Abby","Brenda","Cindy","Diddy" ] for item in items: print(item) |
The for loop can be used to repeat N times too:
#!/usr/bin/python for i in range(1,10): print(i) |
While loop
If you are unsure how many times a code should be repeated, use a while loop.
For example,
correctNumber = 5 guess = 0 while guess != correctNumber: guess = int(input("Guess the number: ")) if guess != correctNumber: print('False guess') print('You guessed the correct number') |
Nested loops
We can combine for loops using nesting. If we want to iterate over an (x,y) field we could use:
#!/usr/bin/python for x in range(1,10): for y in range(1,10): print("(" + str(x) + "," + str(y) + ")") |
Nesting is very useful, but it increases complexity the deeper you nest.
Python range
The range() function returns of generates a sequence of numbers, starting from the lower bound to the upper bound.
range(lower_bound, upper_bound, step_size) |
- lower_bound: The starting value of the list.
- upper_bound: The max value of the list, excluding this number.
- step_bound: The step size, the difference between each number in the list.
The lower_bound and step_size parameters are optional. By default the lower bound is set to zero, the incremental step is set to one. The parameters must be of the type integers, but may be negative.
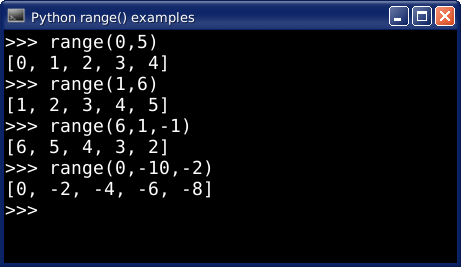
Related Course:
Python Programming Bootcamp: Go from zero to hero
range implementation difference
This distinction won’t usually be an issue. The range() is implemented slightly different in the Python versions:
- Python 2.x: The range() function returns a list.
- Python 3.x: The range() function generates a sequence.
range in python 2.7
A call to range(5) will return: 0,1,2,3,4.
>>> range(5) |
A call to range(1,10) returns: 1,2,3,4,5,6,7,8,9
>>> range(1,10) |
Calling range(0,10,2) returns: 0,2,4,6,8
>>> range(0,10,2) |
range in python 3
To generate a list using range, add the list function
>>> list(range(5)) |
We can use all parameters (lower bound, upper bound, step)
>>> list(range(0,10,2)) [0, 2, 4, 6, 8] |
python 2 implementation
This version of range() allocates computer memory and also populates the computer memory behind the scenes. For large ranges, this is implementation is not very efficient.
Usually you won’t have any issues with the Python2 implementation of range() but if you use large numbers (millions of items) you could run into issues.
Python tuple
The tuple data structure is used to store a group of data. The elements in this group are separated by a comma. Once created, the values of a tuple cannot change.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Python Tuple
An empty tuple in Python would be defined as:
tuple = () |
A comma is required for a tuple with one item:
tuple = (3,) |
The comma for one item may be counter intuitive, but without the comma for a single item, you cannot access the element. For multiple items, you do not have to put a comma at the end. This set is an example:
personInfo = ("Diana", 32, "New York") |
The data inside a tuple can be of one or more data types such as text and numbers.
Data access
To access the data we can simply use an index. As usual, an index is a number between brackets:
#!/usr/bin/env python personInfo = ("Diana", 32, "New York") print(personInfo[0]) print(personInfo[1]) |
If you want to assign multiple variables at once, you can use tuples:
#!/usr/bin/env python name,age,country,career = ('Diana',32,'Canada','CompSci') print(country) |
On the right side the tuple is written. Left of the operator equality operator are the corresponding output variables.
Append to a tuple in Python
If you have an existing tuple, you can append to it with the + operator. You can only append a tuple to an existing tuple.
#!/usr/bin/env python x = (3,4,5,6) x = x + (1,2,3) print(x) |