python socket
Python hosting: Host, run, and code Python in the cloud!
In this tutorial you will learn about in network programming. You will learn about the client-server model that is in use for the World Wide Web, E-mail and many other applications.
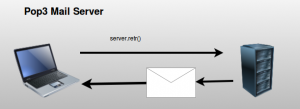
Related course:
If you prefer a course or certification:
socket server code
This code will start a simple web server using sockets. It waits for a connection and if a connection is received it will output the bytes received.
#!/usr/bin/env python |
Execute with:
$ python server.py |
This opens the web server at port 62. In a second screen, open a client with Telnet. If you use the same machine for the client and server use:
$ telnet 127.0.0.1 62. |
If you use another machine as client, type the according IP address of that machine. You can find it with ifconfig.
Everything you write from the client will arrive at the server. The server sends the received messages back. An example output below (Click to enlarge):
socket network client:
The client script below sends a message to the server. The server must be running!
#!/usr/bin/env python |
This client simply mimics the behavior we did in Telnet.
Limitations of the server code
The server code above can only interact with one client. If you try to connect with a second terminal it simply won’t reply to the new client. To let the server interact with multiple clients you need to use multi-threading. We rebuild the server script to accept multiple client connections:
#!/usr/bin/env python |
Application protocol
So far we have simply sent messages back and forth. Every message can have a specific meaning in an application. This is known as the protocol. The meaning of these messages must be the same on both the sender and receiver side. The Transport Layer below makes sure that messages are received (TCP). The Internet Layer is the IPv4 protocol. All we have to define is the Application Layer.
Below we modified the server to accept simple commands (We use the non-threading server for simplicity). We changed the port to 64. Server code with a protocol:
#!/usr/bin/env python |
Run the server with:
sudo python server.py |
A client can then connect with telnet (make sure you pick the right IP):
$ telnet 127.0.0.1 64 |
Leave a Reply:
What is the difference between Web programming and network programming?
Compared to the human communication: The web is a language on top of sockets, this computer language is called 'http'. A network programming program can speak any language ('http','email','ftp','irc'..). "Web programming : network" is as "English : sounds"
Thank you!I am a Chinese,could I follow you on twitter?谢谢!
Yes, https://twitter.com/pythonspot