qpushbutton signals
Python hosting: Host, run, and code Python in the cloud!
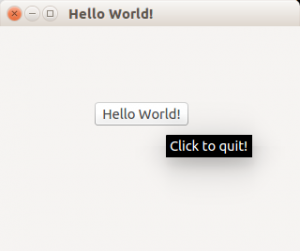
PyQt4 (Qt4) supports buttons through the QPushButton widget.
We extend the code to display a button in the center of the window.
The button will show a tooltip if hovered and when pressed will close the program.
Related course:
PyQt4 button example
The example below adds a button to a PyQt4 window.
|
PyQt4 signals and slots
A button click should do something. To do so, you must use signals and slots.
If a user does an action such as clicking on a button, typing text in a box – the widget sends out a signal. Signals can be connected with a slot, that acts as a receiver and acts on it.
Note that these days, PyQt5 can be used to create a qpushbutton. Then use signals and slots to make it interactive.
|
Posted in qt4
Leave a Reply:
hi that is great tutorial, I have a question ,I do not understand why I use QtCore , I write code same in my idle and I delete part @pyqtSlot() , so it work correctly again.
These are decorators, but in this case not necessary.
okay , thank you Frank