Tag: matplot
Matplotlib legend
matplotlib save figure
matplotlib update plot
Updating a matplotlib plot is straightforward. Create the data, the plot and update in a loop.
Setting interactive mode on is essential: plt.ion(). This controls if the figure is redrawn every draw() command. If it is False (the default), then the figure does not update itself.
Update plot example
Copy the code below to test an interactive plot.
|
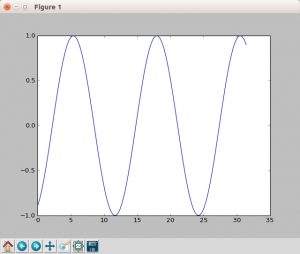
Explanation
We create the data to plot using:
|
Turn on interacive mode using:
|
Configure the plot (the ‘b-‘ indicates a blue line):
|
And finally update in a loop:
|
matplotlib time axis
Matplotlib supports plots with time on the horizontal (x) axis. The data values will be put on the vertical (y) axis. In this article we’ll demonstrate that using a few examples.
It is required to use the Python datetime module, a standard module.
Related course
Plot time
You can plot time using a timestamp:
|
If you want to change the interval use one of the lines below:
|
Time plot from specific hour/minute
To start from a specific date, create a new timestamp using datetime.datetime(year, month, day, hour, minute).
Full example:
|
matplotlib heatmap
A heatmap can be created using Matplotlib and numpy.
Related courses
If you want to learn more on data visualization, this course is good:
Heatmap example
The histogram2d function can be used to generate a heatmap.
We create some random data arrays (x,y) to use in the program. We set bins to 64, the resulting heatmap will be 64x64. If you want another size change the number of bins.
|
Result:
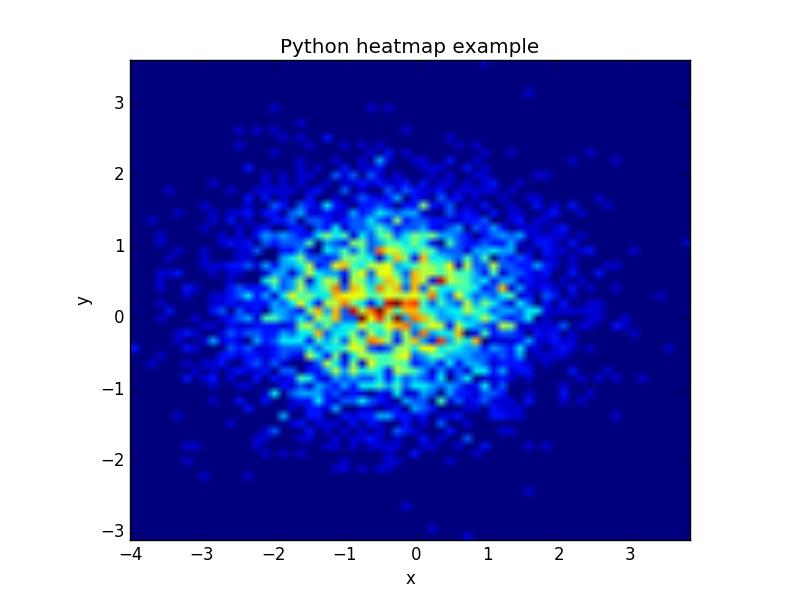
The datapoints in this example are totally random and generated using np.random.randn()