Tag: wxpython
wxPython window
wxpython button
To create a button simply call wx.Button(). When creating a button with wx.Button() it is important to parse the panel as first argument. We attach it to a panel because attaching to the frame would make it full screen.
A panel gives you to option to position widgets anywhere in the window. The parameter (10,10) is the position on the panel. The id argument is necessary, but it is equal to -1 (wx.ID_ANY == -1). The 3rd parameter is the text on the button.
Related course:
Creating GUI Applications with wxPython
You can use the code below to create a button in wxPython:
#!/usr/bin/python |
The function onButton() is called if the button is pressed. We bind (connect) it with button.Bind(wx.EVT_BUTTON, onButton).
Output:
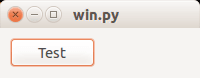
Image on button
wxPython supports having images on buttons. Only a minor change is needed to display an image on a button. While the function is called wx.BitmapButton, it supports other image formats.
bmp = wx.Bitmap("call-start.png", wx.BITMAP_TYPE_ANY) |
The first line loads the image, the second line creates the button.
Full code:
#!/usr/bin/python |
Output:
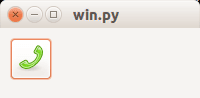
Related course:
Creating GUI Applications with wxPython
wxpython dialog
In this tutorial, you’ll learn how to display various types of dialogs using wxPython, a popular GUI toolkit for Python.
Dialogs play an essential role in user interaction, informing users or seeking confirmation on certain operations. With wxPython, showing these dialogs is straightforward.
Related Course: Creating GUI Applications with wxPython
Information Dialog in wxPython
An information dialog is a simple way to convey messages to users. Here’s how you can display one using wxPython:
1 | import wx |
The first parameter is the message text, the second specifies the dialog title, and the last one defines the dialog’s behavior and appearance.
Other wxPython Dialogs: Warning, Error, and Default
By tweaking the parameters, you can also create other dialog types. Let’s look at a few examples:
1 | import wx |
Creating a Question Dialog in wxPython
wxPython also allows for creating question dialogs, which can be used to seek yes/no responses from users:
1 | import wx |
Once again, remember that wxPython makes it convenient to create and manage GUI applications. The dialogs shown are just a small part of its capabilities.
Learn More: Creating GUI Applications with wxPython
Navigate the tutorial: [
wx python
While working with wxPython, it’s essential to grasp object orientation even if it hasn’t been the main focus of our series. This guide will help you understand how to design a tab interface with wxPython.
Recommended Course: Creating GUI Applications with wxPython
The Mainframe class constructs the frame, similar to previous examples. Other classes serve as the contents for the tabs. Within the main frame, we establish a panel and a notebook (which acts as the tab holder). Following that, we initiate tab objects:
tab1 = TabOne(nb) |
We then attach these tab objects to the tab holder:
nb.AddPage(tab1, "Tab 1") |
Here’s the complete code for better clarity:
import wx |
Output Image:
Enrich your knowledge with: Creating GUI Applications with wxPython