chart flask
Python hosting: Host, run, and code Python in the cloud!
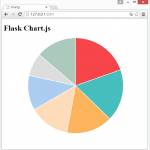
Flask webapp with pie chart
In this article you will learn how to create great looking charts using Chart.js and Flask.
Chart.js is a javascript library to create simple and clean charts. All of them are HTML5 based, responsive, modular, interactive and there are in total 6 charts.
Related course
Python Flask: Make Web Apps with Python
Create the directory /static/ and add the file Chart.min.js to it. You can get it either from the Chart.js website or use the link. Finally go into the home directory and create app.py with this contents:
Chart.js is a javascript library to create simple and clean charts. All of them are HTML5 based, responsive, modular, interactive and there are in total 6 charts.
Related course
Python Flask: Make Web Apps with Python
<meta charset="utf-8"> <title>Chart.js </title> <!-- import plugin script --> <script src="static/Chart.min.js"></script> |
Create the directory /static/ and add the file Chart.min.js to it. You can get it either from the Chart.js website or use the link. Finally go into the home directory and create app.py with this contents:
from flask import Flask |
Finally run:
python app.py |
Open http://127.0.0.1:5001/ and you will see the array values[] plotted with the data in labels[].
We simply pass these two arrays to render_template(). This means that most of the magic occurs in the template. Chart.js is a client-side javascript library which is why our app.py is very minimal.
Result:
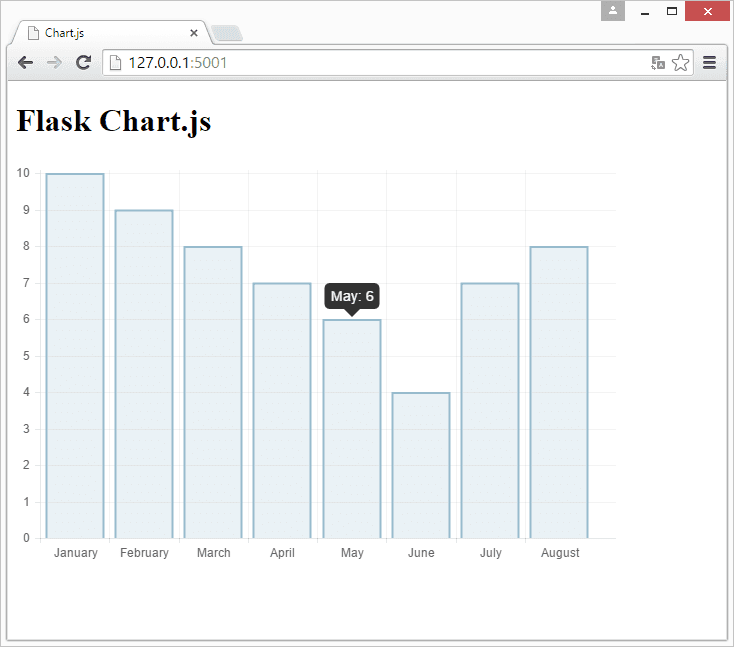
Related course
Python Flask: Make Web Apps with Python
Create a line chart with Chart.js and Flask
To create a line chart, we can simply modify the chart.html template. Change it to:
<meta charset="utf-8"> |
Output:
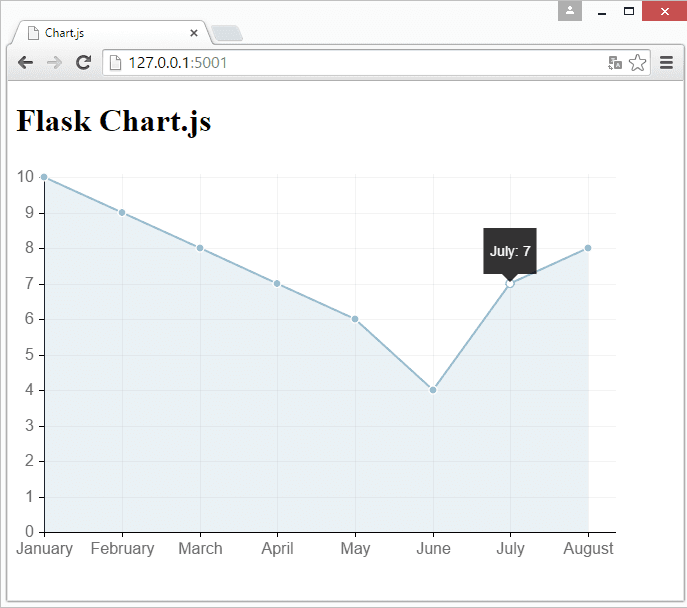
Related course
Python Flask: Make Web Apps with Python
Creating a pie chart
To create a pie chart, we must modify the application code slightly. We need 3 arrays: values, labels and colors. Colors are defined in hexadecimal, as usual in HTML. To iterate them in one loop, we zip them.
from flask import Flask |
Secondly we modify the template to:
<meta charset="utf-8"> <title>Chart.js </title> <!-- import plugin script --> <script src="static/Chart.min.js"></script> |
Result:
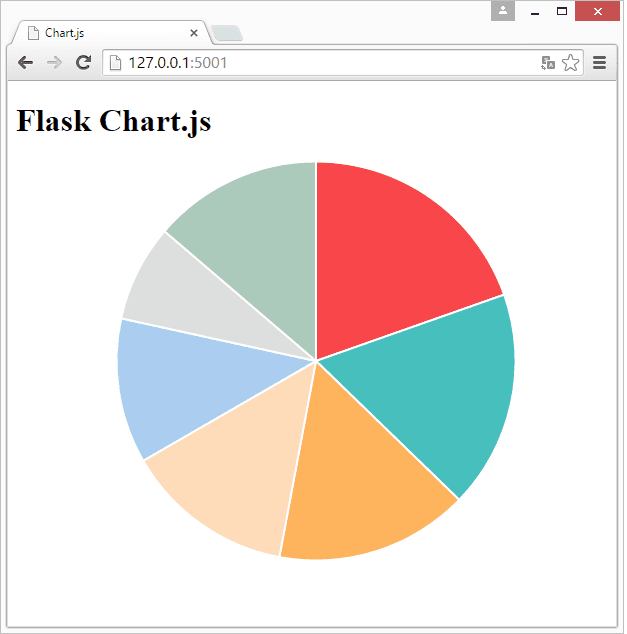
Leave a Reply:
It's a bad idea to use templating for passing data into js code (aka filling gaps). Maybe it's easier to implement but you should make some ajax call to corresponding route on the server to fetch the data. That gives you more flexibility when switching backend/frontend to other technology and avoids mixing jinja2/javascript.
Thanks Misha! That would be more flexible.
I just stumbled across this post a couple weeks ago and thought the method seemed sane (as a JS initiate). Why do you call it filling gaps? I was able to further improve the code (IMHO) by removing the loops from the template by using the tojson filter on the variables directly. It seems to be a fairly straightforward and clean application of that method for use on mostly static data.
I'm hoping that Misha sees my reply, but in the interim and as I mentioned in my reply to him, I was able to further simplify the template by using the tojson filter. Simply replace your loops with, for example:
labels: {{ labels|tojson|safe }},
...
data: {{ values|tojson|safe }},
Thoughts?
Can anyone point to an example or provide some demo code that will do this ajax call? Sorry I am very new to javascript.