python maze game
Python hosting: Host, run, and code Python in the cloud!
In this tutorial you will learn how to build a maze game. The idea is simply to move around the maze with the arrow keys.
Related course:
Create Space Invaders with Python
Getting started: Basic structure and event handling.
We define a class Player which holds the players position on the screen and the speed by which it moves. In addition we define the actions a Player instance can do (movements):
class Player: |
A player object can be created and variables can be modified using the movement methods. We link those methods to the events. In Pygame we can get non-blocking keyboard input using this code:
pygame.event.pump() |
The complete code gives us the ability to move the player across the screen:
from pygame.locals import * |
You can now move the block around the screen with the arrow keys.
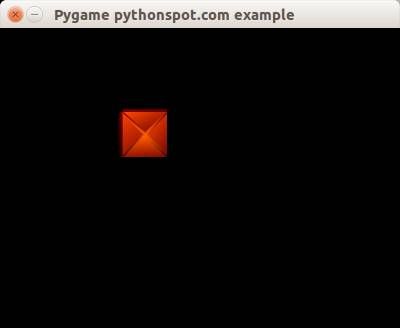
Creating the maze
We define a matrix of NxM to represent the positions of the maze blocks. In this matrix the element 1 represents the presence of a block and element 0 represents the absence.
class Maze: |
We have this complete code to draw the maze:
from pygame.locals import * |
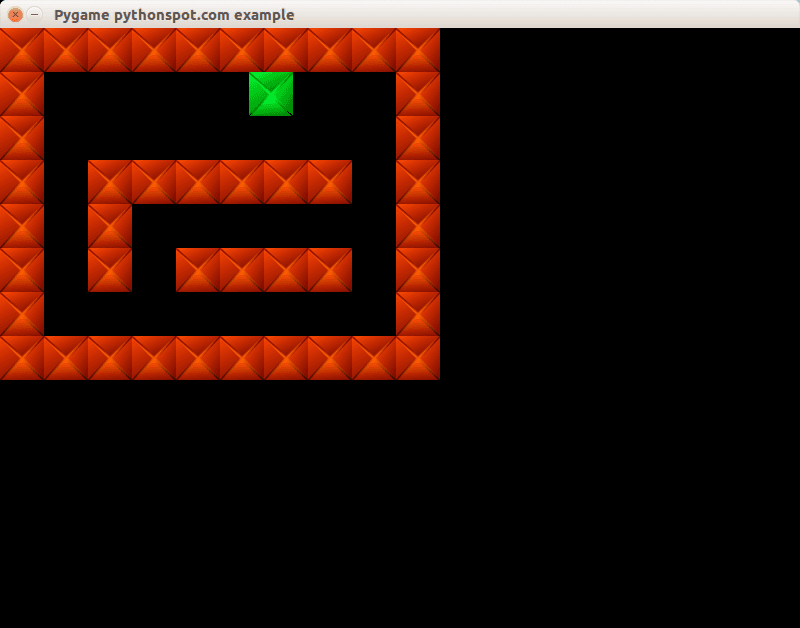
Related course:
Create Space Invaders with Python
Concluding
You learned how to create a 2d maze in Python. Now you may want to add collision detection which we showed in our previous tutorial. Because we already explained that concept we will not go over it again :-)
Leave a Reply:
I'm really not understand in part this:
I'm using Python 3++ sir.and how make this maze and player is solid? please explain it.
Its a one dimensional loop, filling a two dimensional space. To do so, the y position must be updated (+1) once the width is reached.
the matrix self.maze defines which are solid and space. If a cell is '1', its solid
I did not understand these lines of code.
Some syntactical error in these lines. So, please correct it.
This is how the program walks through the screen. It starts at top left and ends on bottom right.
If the maximum horizontal steps are taken (self.M-1), it takes a vertical step.
It goes through the matrix that way. The variables bx and by are the current position in the matrix.
This position changes because its inside a for loop.
Where are the image files 'pygame.png' 'player.png' and 'block.png' to be found?
Just pick any images from the web. I got the cube images from an open source game, but I don't remember which.
Thank you so much for this very useful tutorial !