save dictionary python
Python hosting: Host, run, and code Python in the cloud!
How to make python save a dictionary to a file. These are small programs that allows you to create a dictionary and then, when running the program, it will create a file that contains the data in the original dictionary.
Given a dictionary such as:
|
We can save it to one of these formats:
- Comma seperated value file (.csv)
- Json file (.json)
- Text file (.txt)
- Pickle file (.pkl)
You could also write to a SQLite database.
Related Course:
save dictionary as csv file
The csv module allows Python programs to write to and read from CSV (comma-separated value) files.
CSV is a common format used for exchanging data between applications. The module provides classes to represent CSV records and fields, and allows outputs to be formatted as CSV files.
In this format every value is separated between a comma, for instance like this:
Python,py,programming, |
You can write it to a file with the csv module.
# load csv module |
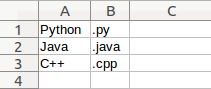
save dictionary to json file
Today, a JSON file has become more and more common to transfer data in the world. JSON (JavaScript Object Notation) is a lightweight data-interchange format.
JSON is easy for humans to read and write. It is easy for machines to parse and generate.
JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
JSON was originally derived from the JavaScript scripting language, but it is not limited to any one programming language.
If you want to save a dictionary to a json file
|
save dictionary to text file (raw, .txt)
The program below writes a dictionary to an text string. It uses the str() call to convert the dictionary to a text string. While it is easy to write as a text string, this format makes it harder to read the file.
You can save your dictionary to a text file using the code below:
# define dict |
save dictionary to a pickle file (.pkl)
The pickle module may be used to save dictionaries (or other objects) to a file. The module can serialize and deserialize Python objects.
In Python, pickle is a built-in module that implements object serialization. It is both cross-platform and cross language, meaning that it can save and load objects between Python programs running on different operating systems, as well as between Python running on different platforms.
The pickle module is written entirely in Python, and is available in CPython implementations, such as Jython or IronPython. To enable the loading of pickles in other Python modules, pickle supports being executed from the command line.
The program below writes it to a pickle file.
|
Related Course: Python Crash Course: Master Python Programming
Leave a Reply: