Category: uncategorized
save dictionary python
python string methods
python find in array
python pause for time
Speech engines with python tutorial
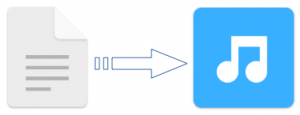
A computer system used to create artificial speech is called a speech synthesizer, and can be implemented in software or hardware products.
A text-to-speech (TTS) system converts normal language text into speech. How can we use speech synthesis in Python?
Related courses:Pyttsx
Pyttsx is a cross-platform speech (Mac OSX, Windows, and Linux) library. You can set voice metadata such as age, gender, id, language and name. Thee speech engine comes with a large amount of voices.
Text to speech sample:
Install with:
|
Create the code speech1.py
|
And execute it with python.
Espeak
eSpeak is a compact open source software speech synthesizer for English and other languages, for Linux and Windows.
Text to speech sample:
We can install using:
|
Create the code speech2.py:
|
It is very easy to use, but like pyttsx it sounds very robotic.
gTTS
The gtts module no longer works.
I found a script on Github that uses the Google speech engine. The script comes with many options and does not speak, instead it saves to an mp3. We added a command to play the mp3 automatically:
|
Run with:
|
The voice is extremely natural. The only disadvantage is that you need to be connected with the Internet when running this script.
Links
You might like:
string methods python
Methods can be applied to Python strings, where a string is somet text between quotes. An example of using the string method replace, which replaces the a sub-string in a string:
|
Related Course:
Python Programming Bootcamp: Go from zero to hero
Overview
Python includes these useful methods for string manipulation:
Method with description | |||||
---|---|---|---|---|---|
capitalize(str) Returns first letter capitalized string. |
|||||
count(str) Count the frequency of the word str. It can also be applied to words: s.count("Hello") |
|||||
find(str) Find the position of the first occurence. |
|||||
index(str) Find the position of the first occurence, raise exception if not found. |
|||||
isdecimal() Returns true if string is decimal. |
|||||
isdigit() Returns true if string contains digits only. |
|||||
isnumeric() Returns true if string contains numeric only. |
|||||
lower() Returns string in lowercase. |
|||||
len(str) Returns the length of str. |
|||||
upper() Returns string in uppercase. |
|||||
replace(old, new) Replaces the first argument with the second argument. |
IDE | Author | Platform | Description | Price | Download |
---|---|---|---|---|---|
PyCharm | Jetbrains | Windows, Mac OS X, Linux/UNIX | Python IDE. Features including: code completion, code inspections, on-the-fly error highlighting and quick-fixes | € 89 / 1st year.($ 97.90) | Download PyCharm |
Atom (+script plugin) | GitHub | Windows, Mac OS X, Linux/UNIX | Python IDE. You need to download the script plugin after installing Atom. | Free. | Download Atom. |
Pythonista | omz:software | Apple iOS (iPhone, iPad) | Features include: Syntax highlighting, code completion, interactive prompt, standard and iOS modules. | € 9. ($ 9.90) | Download Pythonista. |
Eclipse with PyDev | Aleks Totic | Windows, Mac OS X, Linux/UNIX | Features include: Syntax highlighting, code refactoring, graphical debugging and more. | Free | Download |
Eric Python IDE | Detlev Offenbach | Windows, Linux/UNIX | Features include: Syntax highlighting, autocompletion, class browser and more. | Free | Download |
Wing IDE | Wingware | Windows, Mac OS X, Linux/UNIX | Features: Syntax highlighting, auto completion, refactoring, unit testing and version control. | $45 to $245 per user / license. | Download |
Komodo IDE | Komodo | Windows, Mac OS X, Linux/UNIX | Features: Syntax highlighting, documentation browser, run code in-line, quick bookmarks and more. | € 40 to € 223. ($99 to $295). | Download |
Repl.it | Amjad Masad, Haya Odeh, Faris Masad and Max Shawabkeh. | Web | Python interpreter | Free | Run Online |
Ideone | Ideone | Web | Python interpreter | Free | Run Online |
Codepad | Steven Hazel | Web | Python interpreter | Free | Run Online |
|
Using it is as simple as:
|
Complete program
The program below will answer spoken questions.
|
Related posts:
gui in python
To create a graphical interface (GUI) in Python you need to make use of a library or module. There are at least three widely used modules for creating GUIs with Python:
Related course:
GUI toolkits
Tk provides basic widgets such as a button, menu, text and label. It is very limited compared to QT4 and WxPython but it is also the oldest module. It runs on most versions of Mac OS, Linux and Windows.
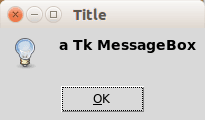
QT4 and QT5 are developed by the Qt company. Graphical applications made with QT4 or QT5 run on Windows, Linux/X11 and Mac OS X. It has tons of widgets including tabs, buttons, item views, table views, progressbars, input fields, calendar views and many more. Compared to Tk it has a lot more widgets available.
WxPython is a module that creates a native GUI look regardless of the operating system used. On Windows it will look as windows application while on Mac OS it will look as a Mac application. This can be a clear advantage compared to QT4 or Tk, depending on your purpose. WxWidgets has many widgets available such as buttons, menu, text but also more advanced widgets as a htmlview or tree control.
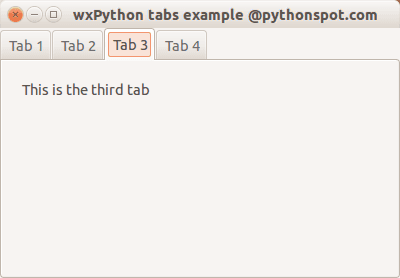