Category: beginner
Python hosting: Host, run, and code Python in the cloud!
Python Debugging
We can use debugging tools to minimize and find bugs. In this article you will learn the best Python debugging tricks.
PuDB – A console-based Python debugger
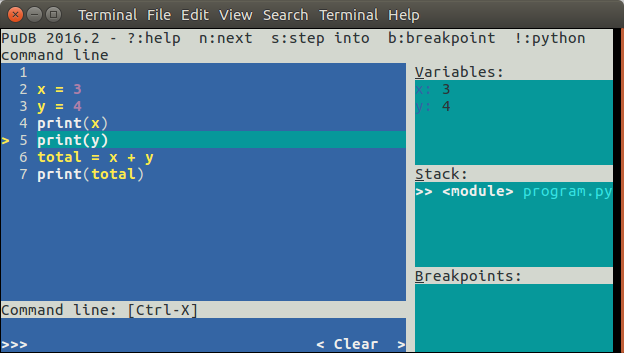
A graphical interface is shown using the PuDB terminal.
Related course:
Python Programming Bootcamp: Go from zero to hero
Installation
to install with Python 3:
sudo pip3 install pudb |
for Python 2.x use:
sudo pip install pudb |
Debugging
Start debugging with:
$ pudb3 program.py |
(or sudo if you don’t have the right permissions)
You can take step by step through the program. Use the n key to take a step through the program. The current variable contents are shown on the right top.
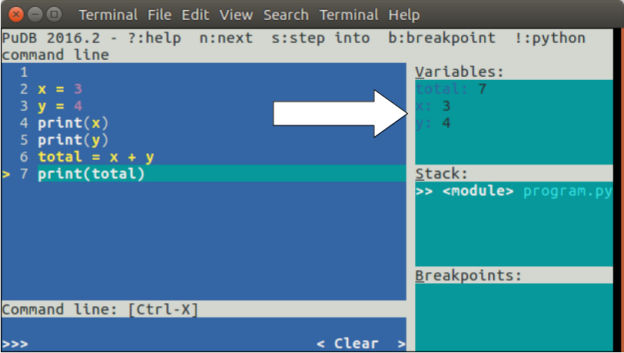
You can set breakpoints using the b key. To continue execution until the next breakpoints, press the c key.
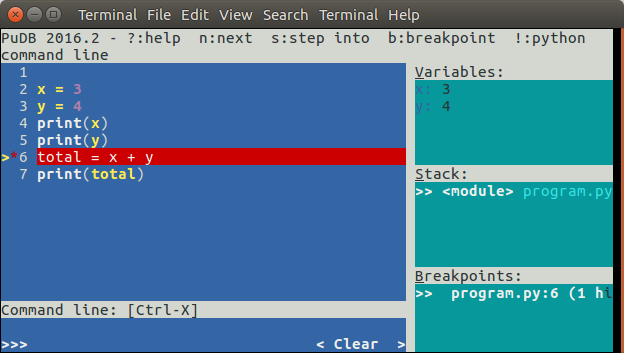
PDB – The Python Debugger
The module pdb supports setting breakpoints. A breakpoint is an intentional pause of the program. where you can get more information about the programs state.
To set a breakpoint, insert the line
pdb.set_trace() |
Example
A practical example:
import pdb x = 3 y = 4 pdb.set_trace() total = x + y pdb.set_trace() |
We have inserted a few breakpoints in this program. The program will pause at each breakpoint (pdb.set_trace()). To view a variables contents simply type the variable name:
$ python3 program.py (Pdb) x 3 (Pdb) y 4 (Pdb) total *** NameError: name 'total' is not defined (Pdb) |
Press c or continue to go on with the programs execution until the next breakpoint
(Pdb) c --Return-- > program.py(7)<module>()->None -> total = x + y (Pdb) total 7 |
Python enum
An enum (enumeration) is a set of symbolic names bound to unique constant values.
We can use enums by referring to their names, as opposed to an index number. The constant value can be any type: numeric or text. As the example below:
currency = enum(EUR='Euro',USD='United States Dollar', GBP='Great British Pound') print(currency.USD) |
Related course
Module enum installation
To use enums module, you need Python 3.4; To install for Python 3.4:
sudo pip install enum34 |
For older versions (Python 2.7):
sudo pip install aenum |
enum example (using enum/aenum)
We can create an Enum after importing the class Enum from either the module enum or aenum.
After importing the list
#from enum import Enum from aenum import Enum class Numbers(Enum): ONE = 1 TWO = 2 THREE = 3 FOUR = 4 print(Numbers.ONE.value) print(Numbers.THREE.value) |
or as a one liner:
#from enum import Enum from aenum import Enum class Numbers(Enum): ONE, TWO, THREE, FOUR = 1,2,3,4 print(Numbers.ONE.value) print(Numbers.THREE.value) |
enum example (without using modules)
The old way of creating enums is:
def enum(**enums): return type('Enum', (), enums) Numbers = enum(ONE=1,TWO=2, THREE=3, FOUR=4, FIVE=5, SIX=6) print(Numbers.ONE) |