pyqt text box
Python hosting: Host, run, and code Python in the cloud!
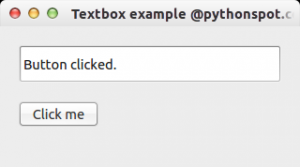
In this article you will learn how to interact with a textbox using PyQt4.
If you want to display text in a textbox (QLineEdit) you could use the setText() method.
Related course:
PyQt4 QLineEdit
The textbox example below changes the text if the button is pressed. Besides text, PyQt can show many more things like images with a qwidget or buttons with qpushbutton.
|
The text field is created with the lines:
|
The button (from screenshot) is made with:
|
We connect the button to the on_click function by:
|
This function sets the textbox using setText().
Posted in qt4
Leave a Reply: