open file python
Python hosting: Host, run, and code Python in the cloud!
In this short tutorial you will learn how to create a file dialog and load its file contents. The file dialog is needed in many applications that use file access.
Related course:
File Dialog Example
To get a filename (not file data) in PyQT you can use the line:
filename = QFileDialog.getOpenFileName(w, 'Open File', '/') |
If you are on Microsoft Windows use
filename = QFileDialog.getOpenFileName(w, 'Open File', 'C:\') |
An example below (includes loading file data):
#! /usr/bin/env python |
Result (output may vary depending on your operating system):
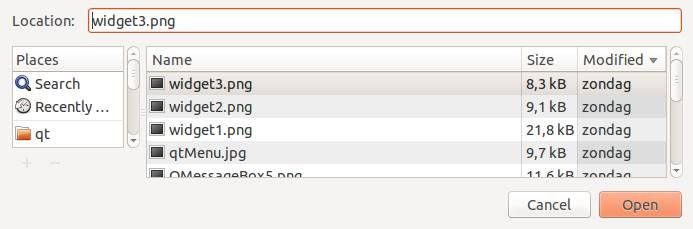
Posted in qt4
Leave a Reply:
Thanks for these great tutorials Frank. They are very helpful!
For windows the line should read:
You can also just do the following to start in current working directory:
It's best to choose an option that is cross platform. Another option is to open the file dialog to the users home directory. Here is a cross platform way of doing that with the Python standard library:
from os.path import expanduser
home_dir = expanduser('~')
Hi there,
I'm somewhat at a loss on labels...how do these work in PYQT4
Thanks
Melissa
To add a label to a window you can call QLabel. I made an example:
How about load an image file and display it?