wxpython
Python hosting: Host, run, and code Python in the cloud!
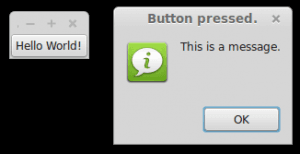
The official wxPython site has several screenshots and downloads for these platforms. wxPython is based on wxWidgets.
Related course: Creating GUI Applications with wxPython
Install wxPython
First download and install WxPython, the Python bindings for wxWidgets.sudo apt-get install python-wxgtk2.8 python-wxtools wx2.8-doc wx2.8-examples wx2.8-headers wx2.8-i18n |
Then install a GUI creator called wxglade:
sudo apt-get install wxglade |
Using a GUI builder such as wxGlade will save you a lot of time, regardless of the GUI library you use. You can easily make complex graphical interfaces because you can simply drag and drop.
Creating our first GUI with Python and wxWidgets:
Start wxglade. You will see its user interface:
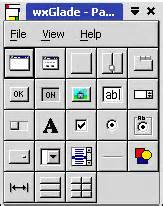
Press on tiny window on the top left, below the file icon.
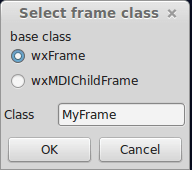
Press OK. An empty window will now appear. Press on the tiny [OK] button in the wxGlade panel and press on the frame. The button will now appear. Press on Application in the tree window.
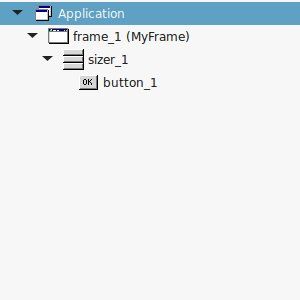
Set the output file in the wxproperties window.
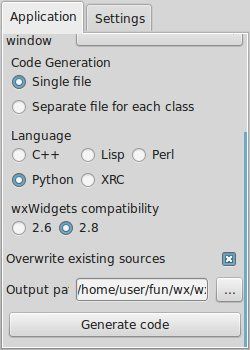
If you look at the window note you can select multiple programming languages and two versions of wxWidgets. Select Python and wxWidgets 2.8. Finally press Generate code. (Do NOT name the file wx.py because the import needs wx, save it as window.py or something else).
Running wxglade code:
Run:
python window.py |
And a window with a button will appear. Pressing the button will not do anything. To start a function when pressing the button, we need to define a so called Callback. This can be as simple as:
def OnButton(self, Event, button_label): |
Finally we bind the button to the callback function using:
self.button_1.Bind(wx.EVT_BUTTON, self.OnButton ) |
Pressing the button will now write a message to the command line. Instead of the boring command line message, we want to show a message box. This can be done using this command:
wx.MessageBox( "This is a message.", "Button pressed."); |
wxPython example code
The full code below:
#!/usr/bin/env python |
Related course: Creating GUI Applications with wxPython
Leave a Reply:
Hello Frank, First of all I find your tutorials very helpful. I installed the wxGlade on my win7 enterprise but when I press the Icon it does nothing. I am using python 2.7.10 fresh install into c:\python27.
I installed wxPython under the c:\python27\lib\site-packeges\wx-3.0-msw and wxglade at the same location under wxGlade. the installation created an icon on the desktop. When I press it nothing happend. Can you help with it ?
Hi Shlomo,
Make sure you have wx runtime installed: https://www.wxwidgets.org/
Is there a log file called wxglade.log in "C:\Users\your username\AppData\wxglade" (or "C:\Users\your username=""\AppData\Roaming\wxglade" ?
Hello Frank. I did the install of all the packages. and at the end I find out that the installer of wxGlade did not created the wxGlade folder under c:\Users\myUserprofile\appdata\roming\wxglade !
I created it manually and now I have the wxGlade SAE working.
I tried to install the SPE IDE and the PyCard Etc in all the cases the same happened it is installed but did not work when pressing the ICON on the desktop. Nor by manually start from the shell.
For now I will work with the already working wxGlade. Later I will check why the others did not work
In any case in your article you should also add installation for Windows not only for linux.