pyqt5 font
Python hosting: Host, run, and code Python in the cloud!
PyQt5 comes with a font dialog that you may have seen in a text editor. This is the typical dialog where you can select font, font size, font style and so on. Of course the look and feel depends on the operating system.
To open this dialog import QFontDialog call:
QFontDialog.getFont() |
To use it, import QFontDialog from QtWidgets like this:
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QFontDialog |
Related course:
PyQt5 font dialog
The example below opens a font dialog on a button event, the selected font (font family including size) will be returned as a string.
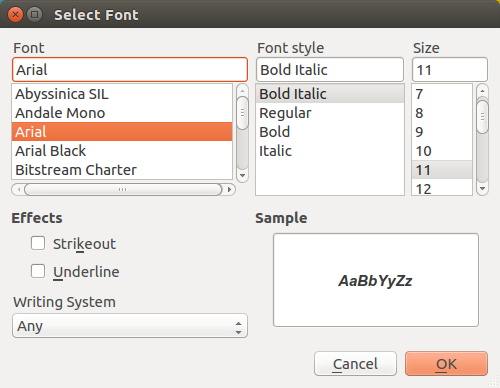
Opening a font dialog is as simple as
def openFontDialog(self): |
Then add a pyqt5 button that opens the dialog. To do that, you need a pyqtslot.
A full code example is shown below
|
If you are new to programming Python PyQt, I highly recommend this book.
Posted in pyqt5
Leave a Reply:
Two typos in the Fonts Dialog example (https://pythonspot.com/pyqt5-font-dialog/):
On line 31, in on_click() 'openFontDialog(self)' should be 'self.openFontDialog()'.
Line 35-36 in openFontDialog() has the wrong indentation. An additional four spaces is needed at the start of each line.
Thanks, updated the code with the right call and indention.
These tutorials have been very helpful, however when I run the above I get 'NameError: name 'ok' is not defined'. how can I correct this?
Seems you have a typo