Car tracking with cascades
Python hosting: Host, run, and code Python in the cloud!
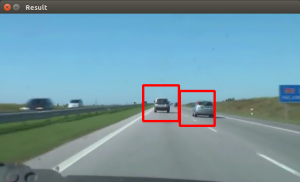
In this tutorial we will look at vehicle tracking using haar features. We have a haar cascade file trained on cars.
The program will detect regions of interest, classify them as cars and show rectangles around them.
Related course:
Master Computer Vision with OpenCV
Detecting with cascades
Lets start with the basic cascade detection program:
#! /usr/bin/python |
This will detect cars in the screen but also noise and the screen will be jittering sometimes. To avoid all of these, we have to improve our car tracking algorithm. We decided to come up with a simple solution.
Related course:
Master Computer Vision with OpenCV
Car tracking algorithm
For every frame:
- Detect potential regions of interest
- Filter detected regions based on vertical,horizontal similarity
- If its a new region, add to the collection
- Clear collection every 30 frames
Removing false positives
The mean square error function is used to remove false positives. We compare vertical and horizontal sides of the images. If the difference is to large or to small it cannot be a car.
ROI detection
A car may not be detected in every frame. If a new car is detected, its added to the collection.
We keep this collection for 30 frames, then clear it.
#!/usr/bin/python |
Final notes
The cascades are not rotation invariant, scale and translation invariant. In addition, Detecting vehicles with haar cascades may work reasonably well, but there is gain with other algorithms (salient points).
You may like:
Leave a Reply:
Good day sir
The algorithm looks quite good and I want to do something similar. May I ask what you used to train the classifier. Did you use some database or did you collect all the training images yourself?
hi, in this case the classifier was already trained with a car image database. If you want to train your own classifier, there are many image databases available freely on computer vision / image processing websites.
Good day, in this case the classifier was already trained with a car image database. If you want to train your own classifier, there are many image databases available freely on computer vision / image processing websites.
Dear Frank,
This is Vijay Shah from High Tech Security Servies, India. We have installed CCTV cameras on highways. Can you give us an application to count & classify vehicles ? We are ready to pay for your development charges.
Hi Vijay, drop me a mail at [email protected]
Hi, I have to track human,cars and buses in the video and count each of them.
Could you please help me how to do that and also how to trained xml for human and buses.
Thanks
There are different techniques to track them. Cascades are one way to track these. OpenCV comes with a bunch of trained cascades. You can find some tutorials here: http://stackoverflow.com/qu...
I'm trying to run your code but I get the following error:
[python]
line 12, in
cars = face_cascade.detectMultiScale(frame, 1.1, 2)
cv2.error: /root/opencv/opencv/modules/core/src/array.cpp:2494: error: (-206) Unrecognized or unsupported array type in function cvGetMat
[/python]
Is it about the array type of frames?
Does it occur on every frame?
The function cvGetMat converts arrays into a Mat. Mat is a datastructure used by cv2 to represent a matrix.
I think there is a file loading error. Do you have cars.xml and video2.avi in the same directory? Make sure the face_cascade and vc are loaded. If loading goes wrong, throw an error.
if x > fwidth*0.4 and x < fwidth*0.5 and y > fheight*0.25 and w > 30 and h > 30: their is syntax error in this cmd
[python]
File "car_detection_original_refinement_new_1.py">car_detection_original_refi...", line 27
if x < fwidth*0.4 and x < fwidth*0.5 and y > fheight*0.25 and w > 30 and h > 30:
^
SyntaxError: invalid syntax
[/python]
how do is solve this.
great post btw
cheers
Try the updated code, make sure the indention is correct. Be sure that you have the cascading file (cars3.xml)
The original repository is no longer available, please check the new link to the cascade file from github.
Try the updated code. Do you have the cascading file (cars.xml) ?
Hello Frank,
Would you mind to share which database you used to train the classifier?
Thank you.
Hi Will,
The classifier is trained using an image database containing cars and non-cars. You can find many of such databases online.
Some datasets:
<ul>
<li>http://ai.stanford.edu/~jkr...
<li>http://archive.ics.uci.edu/...
<li>http://cvlab.epfl.ch/data/p...
</ul>
Hi..How can I track particular car in consecutive frames where I have number of and changing cars in each frame? I need to track and then do some processing on particular detected object.
Hi..I am not getting the way you have calculated ROI using these 0.4 & 0.5 multiplications. Can you please share the logic behind it?
These are simply boundary cases selected chance, you could leave them out. Basically we state that x and y of the top left of the ROI should be in the top left region. Then the sizes of the ROI are checked.
First extract some features (color, structure or salient points) from the target car.
Then detect all cars using cascades and determine if it has these features.
Nice. Very good and excellent tutorial.
However, it seems that the cascade can only detect the car right in front of the camera but not on the right/left track.
Correct, cascades can be trained for certain objects. You could simple download another cascade file from the web, or train yourself.
A left/right cascade:
https://github.com/abhi-kumar/CAR-DETECTION/blob/master/checkcas.xml
Dataset from University of Illinois
http://cogcomp.cs.illinois.edu/Data/Car/
i tried to run this code , its not showing anything , i mean its not showing output , i don't have any errors
Did you download the video + cascade file? Which Python version did you use? Do you have cv2 installed?
[python]
Traceback (most recent call last):
File "C:\Users\ExCITE-LAPTOP\Desktop\Car Tracking\carTracking\detect.py", line 113, in
detectCars('road.avi')
File "C:\Users\ExCITE-LAPTOP\Desktop\Car Tracking\carTracking\detect.py">detect.py", line 93, in detectCars
frameHeight, frameWidth, fdepth = frame.shape
AttributeError: 'NoneType' object has no attribute 'shape'
[/python]
How can I resolve this error? Thanks in Advance
Do you have the files? (road.avi, cars.xml)
Another interesting approach is to use OpenCV HOG + SVM classifier to detect vehicles. Check out this blog:
https://medium.com/@priya.dwivedi/automatic-vehicle-detection-for-self-driving-cars-8d98c086b161#.6x2k4szf5
hi:) Thanks for posting this tut.It helps me to understand a lot. But I got a problem with improved version of your car detection script. The basic script works well (emm...I meant it shows red rectangle when a car is in focus) and your solution doesn't show this red frame. What could be the issue here? Thanks
Is it the same video? The array rectangles is probably empty, inside the loop of detectCars(), check using
[python]
print(len(rectangles))
[/python]
yeap, it's actually empty for some reasons:(
try changing the method isNewRoi, that decides if its added to to the list. The values are set to 40, which is just set by a test. Try changing the values
Dear Sir,
I am facing the below error:
Unable to stop the stream: Inappropriate ioctl for device
Hi Saeed, are you using a webcam or video file?
Frank did you create the xml yourself ?
my openCv training on my Debian serv always stop after stage 0 :(
btw, when i run your last script with Roi the error is :
[python]
frame = cv2.resize(frame, (frameWidth / scaleDown, frameHeight / scaleDown))
TypeError: integer argument expected, got float
[/python]
can you help me with that my friend ?
I don't recall, has been a while. Do you have video capture? Try to show the verify these variables contain the right values using debugger or print function. It mentions a type error, cast frameWidth/scaleDown and the other one to integers using int().
this code is very interresting my friend.
when i do :
frame = cv2.resize(frame, (int(frameWidth / scaleDown), int(frameHeight / scaleDown)))
frameHeight, frameWidth, fdepth = frame.shape
it s say :
left = img[0:height, 0:half]
TypeError: slice indices must be integers or None or have an __index__ method
is it possible to you to write it again or something ? i am just discovering haar cascade and it's very fascinating.
try with both Python 2 and Python 3. Can you display the data beforehand?
Still here my friend ? :)
Hi, has been a few busy days. I've no problem running the code from the zip file.
I'm using Python 2.7.13 on Manjaro Linux.
Did you download the zip file? It has an attached video and haar file.
i did it my friend ;) maybe it's just because i am using Python 3.5 ;)
yeah i did it, but i am running Python 3.5