Category: games
pygame
In this article you will learn how to implement jump and run logic in Pygame. To do so, we will implement the player logic.
You may like
Create Space Invaders with Python
Movement
Moving left and right is similar to the previous tutorial and simply mean changing the (x,y) position of the player. For jumping, we use a formula from classical mechanics:
F = 1/2 * m * v^2 |
Where F is the force up/down, m is the mass of your object and v is the velocity. The velocity goes down over time because when the player jumps the velocity will not increase more in this simulation. If the player reaches the ground, the jump ends. In Python, we set a variable isjump to indicate if the player is jumping or not. If the player is, its position will be updated according to the above formula.
Full Code:
from pygame.locals import * |
If you want to jump on objects, simply add them to the screen, do collision detection and reset the jump variables if collision is true.
You may like
Create Space Invaders with Python
python text game
In this article we will demonstrate how to create a simple guessing game.
The goal of the game is to guess the right number.
Example
An example run below:
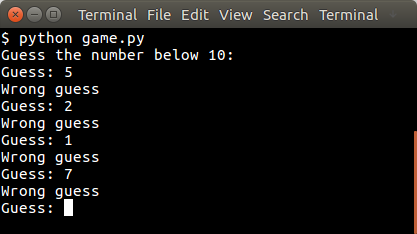
You may like
Simple games with Python
Random number
The user will be asked to guess the random number. We first pick the random number:
from random import randint |
The randint() function will pick a pseudo random number between 1 and 10. Then we have to continue until the correct number is found:
guess = -1 |
Python Guessing Game
The code below starts the game:
from random import randint |
An example run:
Guess the number below 10: |
You may like
Simple games with Python
snake ai
In this article we will show you how to create basic game AI (Artificial Intelligence). This article will describe an AI for the game snake.
In this game (snake) both the computer and you play a snake, and the computer snake tries to catch you. In short: the opponent AI tries to determine and go to the destination point based on your location on the board.
You may like
Adding the computer player:
We extend the code with a new class called Computer which will be our computer player. This contains routines to draw and move the computer snake.
class Computer: |
We then call the update and drawing method of the computer.
def on_loop(self): |
This will make the computer snake move and be drawn on the screen. It has the same properties as the human player.
You may like
Adding the intelligence to the computer player
Because this is a simple game, we do not need to create a complete thinking machine inside the game. We simply need some basic intelligence exhibited by our computer player. Intelligence in games is often quite limited because most of the time more complexity is not neccesary or there simply is not the time available to implement clever algorithms.
The algorithm we will add will simply go to the destination. It will neglect any obstacles (the human player).
def target(self,dx,dy): |
Complete code
We end up with this complete code:
from pygame.locals import * |
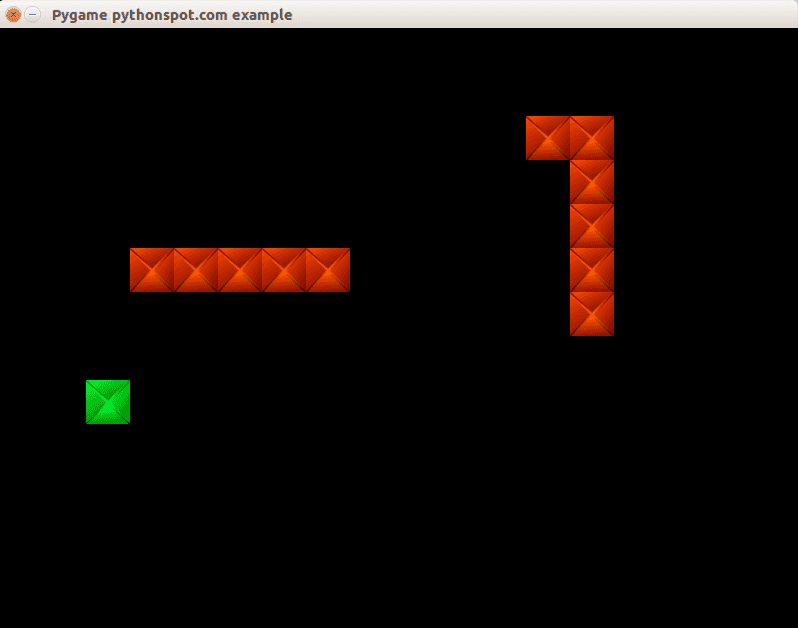
You may like
Conclusion
You learned how to create a basic computer player using an very simple AI algorithm.