Tag: qt
pyqt text box
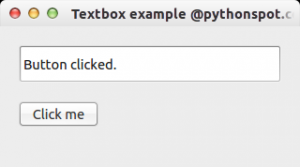
In this article you will learn how to interact with a textbox using PyQt4.
If you want to display text in a textbox (QLineEdit) you could use the setText() method.
Related course:
PyQt4 QLineEdit
The textbox example below changes the text if the button is pressed. Besides text, PyQt can show many more things like images with a qwidget or buttons with qpushbutton.
|
The text field is created with the lines:
|
The button (from screenshot) is made with:
|
We connect the button to the on_click function by:
|
This function sets the textbox using setText().
Download PyQT Code (Bulk Collection)
Qt4 window
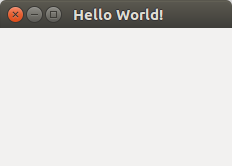
In this tutorial you will learn how to create a graphical hello world application with PyQT4.
PyQT4, it is one of Pythons options for graphical user interface (GUI) programming.
Related course:
PyQt4 window example:
This application will create a graphical window that can be minimized, maximimzed and resized it.
|
The PyQT4 module must be immported, we do that with this line:
|
We create the PyQT4 application object using QApplication():
|
We create the window (QWidget), resize, set the tittle and show it with this code:
|
Don’t forget to show the window:
|
You can download a collection of PyQt4 examples:
Download PyQT Code (Bulk Collection)
qt message box
PyQT4 offers message box functionality using several functions.
Messageboxes included in PyQT4 are: question, warning, error, information, criticial and about box.
Related course: Create GUI Apps with Python PyQt5
PyQt4 mesagebox
The code below will display a message box with two buttons:#! /usr/bin/env python |
Result:
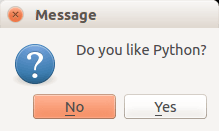
There are different types of messageboxes that PyQT4 provides.
PyQT4 Warning Box
You can display a warning box using this line of code:
QMessageBox.warning(w, "Message", "Are you sure you want to continue?") |
PyQT4 Information box
We can display an information box using QMessageBox.information()
QMessageBox.information(w, "Message", "An information messagebox @ pythonspot.com ") |
Result:
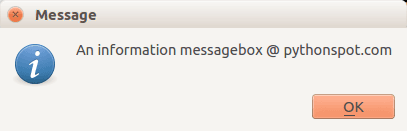
PyQT4 Critical Box
If something goes wrong in your application you may want to display an error message.
QMessageBox.critical(w, "Message", "No disk space left on device.") |
Result:
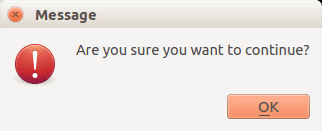
PyQT4 About box
We have shown the question box above.
QMessageBox.about(w, "About", "An example messagebox @ pythonspot.com ") |
Result:
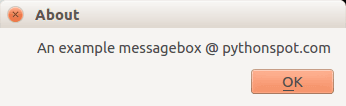
Download PyQT Code (Bulk Collection)
pyqt menu
pyqt widgets
open file python
In this short tutorial you will learn how to create a file dialog and load its file contents. The file dialog is needed in many applications that use file access.
Related course:
File Dialog Example
To get a filename (not file data) in PyQT you can use the line:
filename = QFileDialog.getOpenFileName(w, 'Open File', '/') |
If you are on Microsoft Windows use
filename = QFileDialog.getOpenFileName(w, 'Open File', 'C:\') |
An example below (includes loading file data):
#! /usr/bin/env python |
Result (output may vary depending on your operating system):
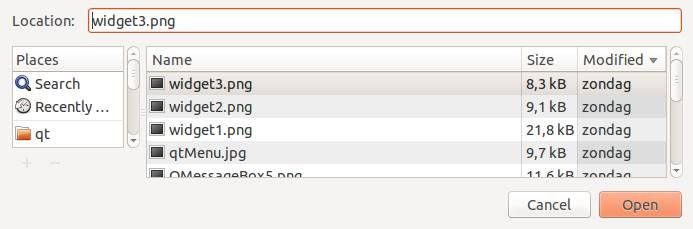