Category: pro
Python hosting: Host, run, and code Python in the cloud!
QT GUI
QT4 Table
We can show a table using the QTableWidget, part of the PyQt module. We set the title, row count, column count and add the data.
Related course:
Qt4 Table example
An example below:
from PyQt4.QtGui import * from PyQt4.QtCore import * import sys def main(): app = QApplication(sys.argv) table = QTableWidget() tableItem = QTableWidgetItem() # initiate table table.setWindowTitle("QTableWidget Example @pythonspot.com") table.resize(400, 250) table.setRowCount(4) table.setColumnCount(2) # set data table.setItem(0,0, QTableWidgetItem("Item (1,1)")) table.setItem(0,1, QTableWidgetItem("Item (1,2)")) table.setItem(1,0, QTableWidgetItem("Item (2,1)")) table.setItem(1,1, QTableWidgetItem("Item (2,2)")) table.setItem(2,0, QTableWidgetItem("Item (3,1)")) table.setItem(2,1, QTableWidgetItem("Item (3,2)")) table.setItem(3,0, QTableWidgetItem("Item (4,1)")) table.setItem(3,1, QTableWidgetItem("Item (4,2)")) # show table table.show() return app.exec_() if __name__ == '__main__': main() |
Result:
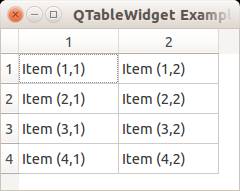
QTableWidget labels
You can set the header using the setHorizontalHeaderLabels() function. The same applies for vertical labels. A demonstration below:
from PyQt4.QtGui import * from PyQt4.QtCore import * import sys def main(): app = QApplication(sys.argv) table = QTableWidget() tableItem = QTableWidgetItem() # initiate table table.setWindowTitle("QTableWidget Example @pythonspot.com") table.resize(400, 250) table.setRowCount(4) table.setColumnCount(2) # set label table.setHorizontalHeaderLabels(QString("H1;H2;").split(";")) table.setVerticalHeaderLabels(QString("V1;V2;V3;V4").split(";")) # set data table.setItem(0,0, QTableWidgetItem("Item (1,1)")) table.setItem(0,1, QTableWidgetItem("Item (1,2)")) table.setItem(1,0, QTableWidgetItem("Item (2,1)")) table.setItem(1,1, QTableWidgetItem("Item (2,2)")) table.setItem(2,0, QTableWidgetItem("Item (3,1)")) table.setItem(2,1, QTableWidgetItem("Item (3,2)")) table.setItem(3,0, QTableWidgetItem("Item (4,1)")) table.setItem(3,1, QTableWidgetItem("Item (4,2)")) # show table table.show() return app.exec_() if __name__ == '__main__': main() |
Result:
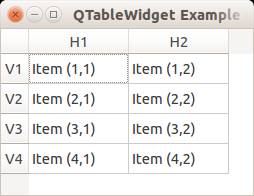
QTableWidget click events
We can detect cell clicks using this procedure, first add a function:
# on click function table.cellClicked.connect(cellClick) |
Then define the function:
def cellClick(row,col): print "Click on " + str(row) + " " + str(col) |
The Python programming language starts counting with 0, so when you press on (1,1) you will see (0,0). Full code to detect table clicks:
from PyQt4.QtGui import * from PyQt4.QtCore import * import sys def cellClick(row,col): print "Click on " + str(row) + " " + str(col) def main(): app = QApplication(sys.argv) table = QTableWidget() tableItem = QTableWidgetItem() # initiate table table.setWindowTitle("QTableWidget Example @pythonspot.com") table.resize(400, 250) table.setRowCount(4) table.setColumnCount(2) # set label table.setHorizontalHeaderLabels(QString("H1;H2;").split(";")) table.setVerticalHeaderLabels(QString("V1;V2;V3;V4").split(";")) # set data table.setItem(0,0, QTableWidgetItem("Item (1,1)")) table.setItem(0,1, QTableWidgetItem("Item (1,2)")) table.setItem(1,0, QTableWidgetItem("Item (2,1)")) table.setItem(1,1, QTableWidgetItem("Item (2,2)")) table.setItem(2,0, QTableWidgetItem("Item (3,1)")) table.setItem(2,1, QTableWidgetItem("Item (3,2)")) table.setItem(3,0, QTableWidgetItem("Item (4,1)")) table.setItem(3,1, QTableWidgetItem("Item (4,2)")) # on click function table.cellClicked.connect(cellClick) # show table table.show() return app.exec_() if __name__ == '__main__': main() |
If you want to show the cell/row numbers in a non-programmer way use this instead:
def cellClick(row,col): print "Click on " + str(row+1) + " " + str(col+1) |
Tooltip text
We can set tooltip (mouse over) text using the method. If you set tooltips on non-existing columns you will get an error.
from PyQt4.QtGui import * from PyQt4.QtCore import * import sys def main(): app = QApplication(sys.argv) table = QTableWidget() tableItem = QTableWidgetItem() # initiate table table.setWindowTitle("QTableWidget Example @pythonspot.com") table.resize(400, 250) table.setRowCount(4) table.setColumnCount(2) # set label table.setHorizontalHeaderLabels(QString("H1;H2;").split(";")) table.setVerticalHeaderLabels(QString("V1;V2;V3;V4").split(";")) # set data table.setItem(0,0, QTableWidgetItem("Item (1,1)")) table.setItem(0,1, QTableWidgetItem("Item (1,2)")) table.setItem(1,0, QTableWidgetItem("Item (2,1)")) table.setItem(1,1, QTableWidgetItem("Item (2,2)")) table.setItem(2,0, QTableWidgetItem("Item (3,1)")) table.setItem(2,1, QTableWidgetItem("Item (3,2)")) table.setItem(3,0, QTableWidgetItem("Item (4,1)")) table.setItem(3,1, QTableWidgetItem("Item (4,2)")) # tooltip text table.horizontalHeaderItem(0).setToolTip("Column 1 ") table.horizontalHeaderItem(1).setToolTip("Column 2 ") # show table table.show() return app.exec_() if __name__ == '__main__': main() |
Result:
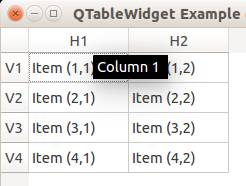
QT4 Tabs
Tabs are very useful in graphical applications. They appear in webbrowsers, text editors and any other apps. To create a tabbed window, you need to call the QTabWidget() function. Every tab is a QWidget() which you have seen before. You can connect the QWidgets with the QTabWidget with the function:
tabs.addTab(tab1,"Tab 1") |
where the first parameter is the tab object and the second the name that appears on the screen. We added some buttons to the first tab (QWidget).
Related course:
Example code:
from PyQt4 import QtGui from PyQt4 import QtCore import sys def main(): app = QtGui.QApplication(sys.argv) tabs = QtGui.QTabWidget() # Create tabs tab1 = QtGui.QWidget() tab2 = QtGui.QWidget() tab3 = QtGui.QWidget() tab4 = QtGui.QWidget() # Resize width and height tabs.resize(250, 150) # Set layout of first tab vBoxlayout = QtGui.QVBoxLayout() pushButton1 = QtGui.QPushButton("Start") pushButton2 = QtGui.QPushButton("Settings") pushButton3 = QtGui.QPushButton("Stop") vBoxlayout.addWidget(pushButton1) vBoxlayout.addWidget(pushButton2) vBoxlayout.addWidget(pushButton3) tab1.setLayout(vBoxlayout) # Add tabs tabs.addTab(tab1,"Tab 1") tabs.addTab(tab2,"Tab 2") tabs.addTab(tab3,"Tab 3") tabs.addTab(tab4,"Tab 4") # Set title and show tabs.setWindowTitle('PyQt QTabWidget @ pythonspot.com') tabs.show() sys.exit(app.exec_()) if __name__ == '__main__': main() |
Result:
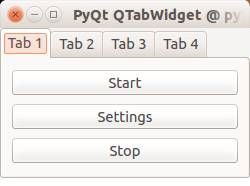
QT4 File Dialog
In this short tutorial you will learn how to create a file dialog and load its file contents. The file dialog is needed in many applications that use file access.
Related course:
File Dialog Example
To get a filename (not file data) in PyQT you can use the line:
filename = QFileDialog.getOpenFileName(w, 'Open File', '/') |
If you are on Microsoft Windows use
filename = QFileDialog.getOpenFileName(w, 'Open File', 'C:\') |
An example below (includes loading file data):
#! /usr/bin/env python # -*- coding: utf-8 -*- # import sys from PyQt4.QtGui import * # Create an PyQT4 application object. a = QApplication(sys.argv) # The QWidget widget is the base class of all user interface objects in PyQt4. w = QWidget() # Set window size. w.resize(320, 240) # Set window title w.setWindowTitle("Hello World!") # Get filename using QFileDialog filename = QFileDialog.getOpenFileName(w, 'Open File', '/') print filename # print file contents with open(filename, 'r') as f: print(f.read()) # Show window w.show() sys.exit(a.exec_()) |
Result (output may vary depending on your operating system):
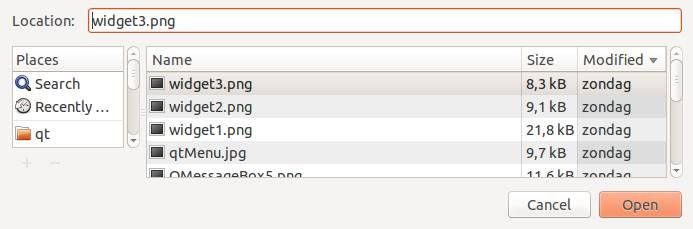
QT4 Pixmaps (Images)
In this article we will demonstrate how to load and display images in an PyQT window. We can display images in a PyQT window using the Pixmap widget.
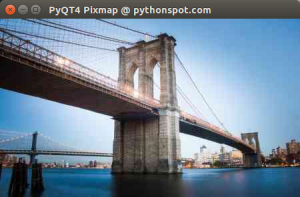
Related course:
Introduction
The constructor of Pixmap takes the image path as parameter:
pixmap = QPixmap(os.getcwd() + 'https://pythonspot-9329.kxcdn.com/logo.png') |
This image needs to be in the same directory as your program. The QPixmap widget supports png and jpeg. Example code below.
PyQT load image in Pixmap
We create a standard QWidget as we have done before. Then we add the QPixmap widget inside which will load the image. The Pixmap is attached to a label which is drawn to the screen.
import os import sys from PyQt4.QtGui import * # Create window app = QApplication(sys.argv) w = QWidget() w.setWindowTitle("PyQT4 Pixmap @ pythonspot.com ") # Create widget label = QLabel(w) pixmap = QPixmap(os.getcwd() + 'https://pythonspot-9329.kxcdn.com/logo.png') label.setPixmap(pixmap) w.resize(pixmap.width(),pixmap.height()) # Draw window w.show() app.exec_() |
Download PyQT Code (Bulk Collection)
Result:
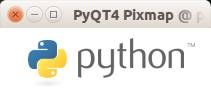
QT4 Progressbar
In this article we will demonstrate how to use the progressbar widget. The progressbar is different from the other widgets in that it updates in time.
Related course:
QT4 Progressbar Example
Let’s start with the code:
#! /usr/bin/env python # -*- coding: utf-8 -*- # import sys from PyQt4.QtGui import * from PyQt4.QtCore import * from PyQt4.QtCore import pyqtSlot,SIGNAL,SLOT class QProgBar(QProgressBar): value = 0 @pyqtSlot() def increaseValue(progressBar): progressBar.setValue(progressBar.value) progressBar.value = progressBar.value+1 # Create an PyQT4 application object. a = QApplication(sys.argv) # The QWidget widget is the base class of all user interface objects in PyQt4. w = QWidget() # Set window size. w.resize(320, 240) # Set window title w.setWindowTitle("PyQT4 Progressbar @ pythonspot.com ") # Create progressBar. bar = QProgBar(w) bar.resize(320,50) bar.setValue(0) bar.move(0,20) # create timer for progressBar timer = QTimer() bar.connect(timer,SIGNAL("timeout()"),bar,SLOT("increaseValue()")) timer.start(400) # Show window w.show() sys.exit(a.exec_()) |
The instance bar (of class QProgBar) is used to hold the value of the progressbar. We call the function setValue() to update its value. The parameter w is given to attach it to the main window. We then move it to position (0,20) on the screen and give it a width and height.
To update the progressbar in time we need a QTimer(). We connect the widget with the timer, which calls the function increaseValue(). We set the timer to repeat the function call every 400 milliseconds. You also see the words SLOT and SIGNAL. If a user does an action such as clicking on a button, typing text in a box – the widget sends out a signal. This signal does nothing, but it can be used to connect with a slot, that acts as a receiver and acts on it.
Result:
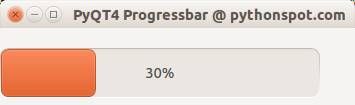
Creating a web-browser with Python and PyQT
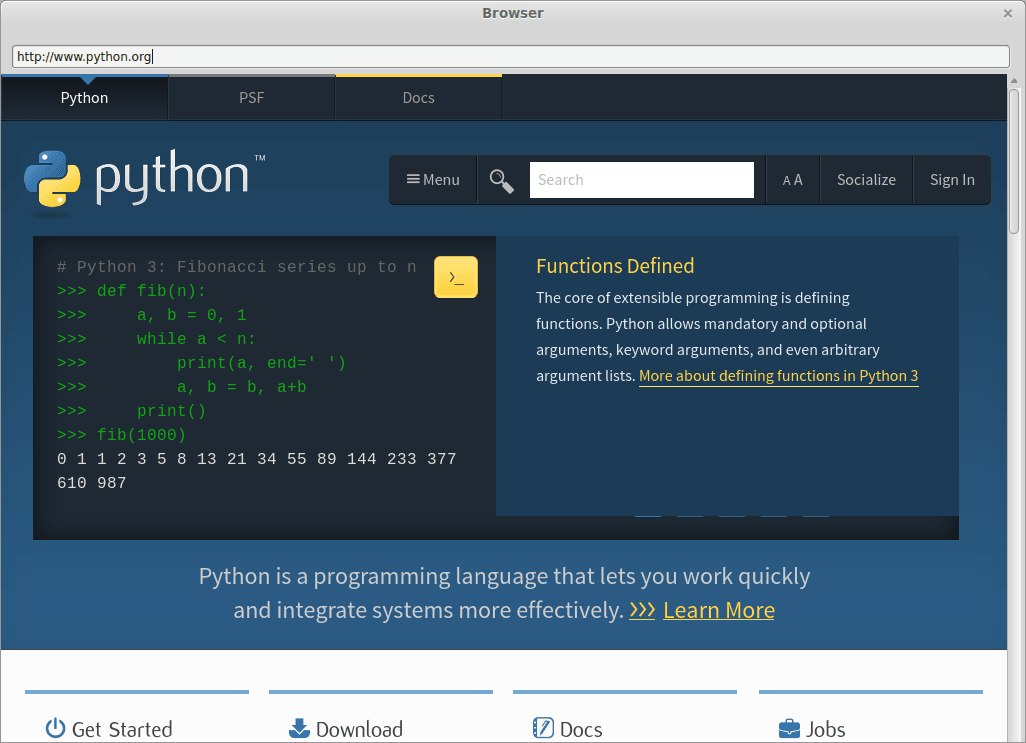
In this tutorial we will build a webbrowser with Python. We will use the PyQT library which has a web component. In this tutorial you will learn how to link all the components together. We will use the default rendering engine and not roll one in this tutorial.
If you have not done our pyqt4 beginner tutorial, you could try it. If python-kde4 cannot be found update your repository to find it. The Ubuntu or Debian install guide .
Related course:
PyQt installation
Install the required packages:
sudo pip install python-qt4 sudo apt-get install qt4-designer sudo apt-get install pyqt4-dev-tools sudo apt-get install python-kde4 |
Creating the GUI with PyQT
Start qt4-designer from your applications menu. The QT Designer application will appear:
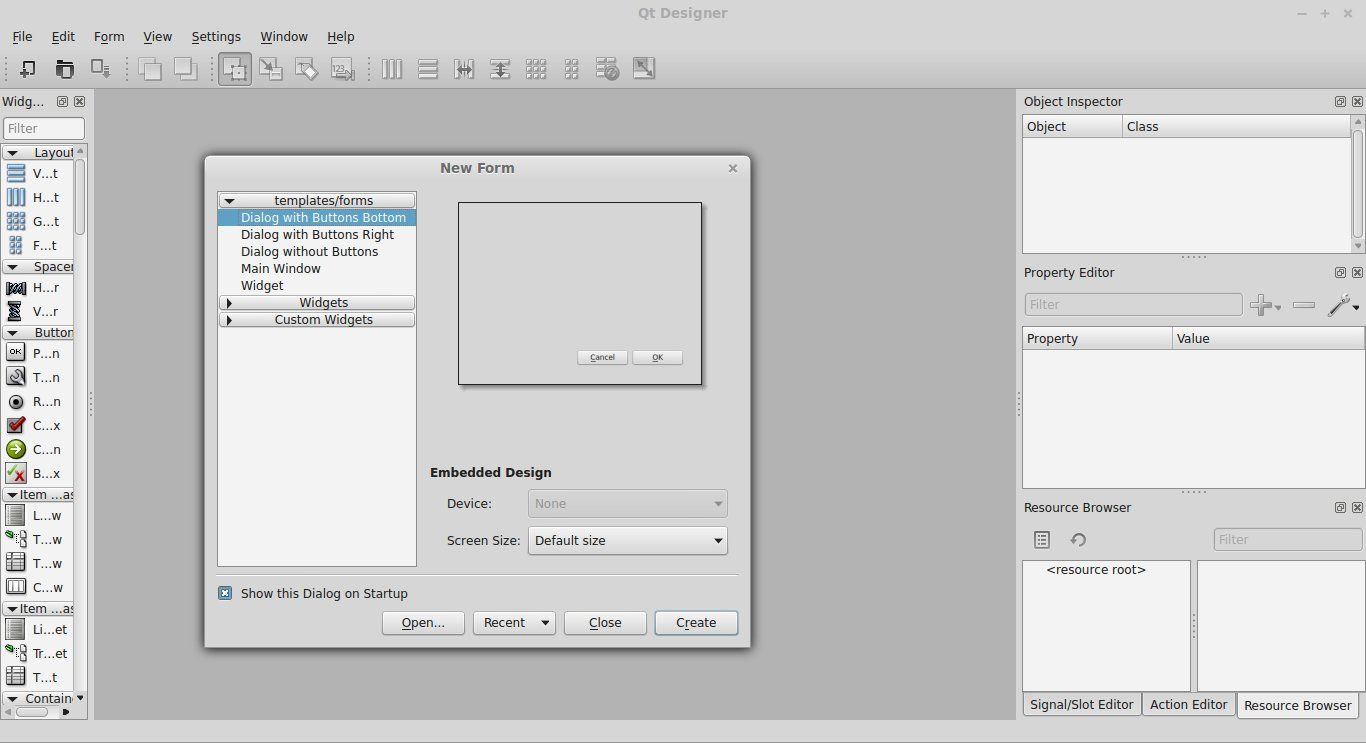
Select Main Window and press Create. We now have our designer window open. Drag a KWebView component on the window. If you have a QtWebView in the component list. use that instead. We also add an Line Edit on top. Press File > Save As > browser.ui. Run the command:
pyuic4 browser.ui > browser.py |
This will generate a Python file. Remove the line “from kwebview import KWebView” from the bottom of the browser.py file. Change KWebView to QtWebView. We want to use QtWebView instead. If you are lazy to change that, take the browser.py file from below.
QWebView exploration
Create a file called run.py with this contents:
import sys from browser import BrowserDialog from PyQt4 import QtCore, QtGui from PyQt4.QtCore import QUrl from PyQt4.QtWebKit import QWebView class MyBrowser(QtGui.QDialog): def __init__(self, parent=None): QtGui.QWidget.__init__(self, parent) QWebView.__init__(self) self.ui = BrowserDialog() self.ui.setupUi(self) self.ui.lineEdit.returnPressed.connect(self.loadURL) def loadURL(self): url = self.ui.lineEdit.text() self.ui.qwebview.load(QUrl(url)) self.show() #self.ui.lineEdit.setText("") if __name__ == "__main__": app = QtGui.QApplication(sys.argv) myapp = MyBrowser() myapp.ui.qwebview.load(QUrl('http://www.pythonspot.com')) myapp.show() sys.exit(app.exec_()) |
This code will use the UI as defined in browser.py and add logic to it. The lines
self.ui.lineEdit.returnPressed.connect(self.loadURL) def loadURL(self): url = self.ui.lineEdit.text() self.ui.qwebview.load(QUrl(url)) self.show() #self.ui.lineEdit.setText("") |
The first line defines the callback or event. If a person presses enter (returnPressed), it will call the function loadURL. It makes sure that once you press enter, the page is loaded with that function. If you did everything correctly, you should be able to run the browser with the command:
python run.py |
Please make sure you type the full url, e.g. : https://pythonspot.com including the http:// part. Your browser should now start:
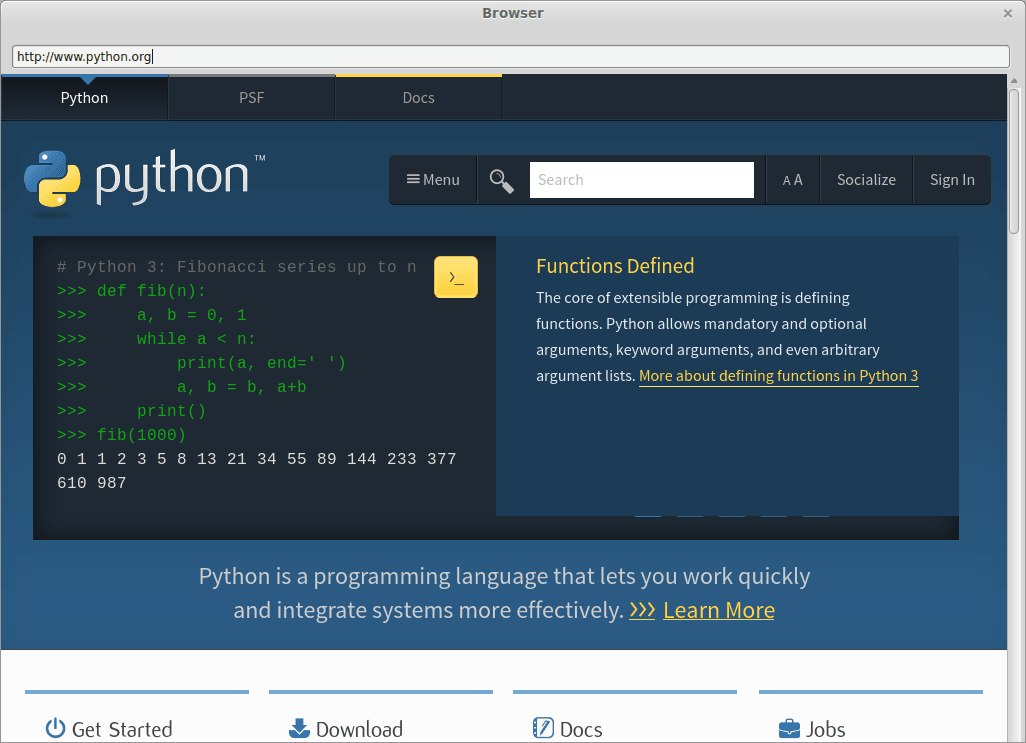
If your code does not run, please use the codes below (or look at the differences and change whats wrong):
browser.py
# -*- coding: utf-8 -*- # Form implementation generated from reading ui file 'browser.ui' # # Created: Fri Jan 30 20:49:32 2015 # by: PyQt4 UI code generator 4.10.4 # # WARNING! All changes made in this file will be lost! import sys from PyQt4 import QtCore, QtGui from PyQt4.QtGui import QApplication from PyQt4.QtCore import QUrl from PyQt4.QtWebKit import QWebView try: _fromUtf8 = QtCore.QString.fromUtf8 except AttributeError: def _fromUtf8(s): return s try: _encoding = QtGui.QApplication.UnicodeUTF8 def _translate(context, text, disambig): return QtGui.QApplication.translate(context, text, disambig, _encoding) except AttributeError: def _translate(context, text, disambig): return QtGui.QApplication.translate(context, text, disambig) class BrowserDialog(object): def setupUi(self, Dialog): Dialog.setObjectName(_fromUtf8("Dialog")) Dialog.resize(1024, 768) self.qwebview = QWebView(Dialog) self.qwebview.setGeometry(QtCore.QRect(0, 50, 1020, 711)) self.qwebview.setObjectName(_fromUtf8("kwebview")) self.lineEdit = QtGui.QLineEdit(Dialog) self.lineEdit.setGeometry(QtCore.QRect(10, 20, 1000, 25)) self.lineEdit.setObjectName(_fromUtf8("lineEdit")) self.retranslateUi(Dialog) QtCore.QMetaObject.connectSlotsByName(Dialog) def retranslateUi(self, Dialog): Dialog.setWindowTitle(_translate("Browser", "Browser", None)) |
run.py
import sys from browser import BrowserDialog from PyQt4 import QtCore, QtGui from PyQt4.QtCore import QUrl from PyQt4.QtWebKit import QWebView class MyBrowser(QtGui.QDialog): def __init__(self, parent=None): QtGui.QWidget.__init__(self, parent) QWebView.__init__(self) self.ui = BrowserDialog() self.ui.setupUi(self) self.ui.lineEdit.returnPressed.connect(self.loadURL) def loadURL(self): url = self.ui.lineEdit.text() self.ui.qwebview.load(QUrl(url)) self.show() #self.ui.lineEdit.setText("") if __name__ == "__main__": app = QtGui.QApplication(sys.argv) myapp = MyBrowser() myapp.ui.qwebview.load(QUrl('http://www.pythonspot.com')) myapp.show() sys.exit(app.exec_()) |