Tag: pyqt
pyqt grid layout
PyQt5 supports a grid layout, which is named QGridLayout. Widgets can be added to a grid in both the horizontal and vertical direction. An example of a grid layout with widgets is shown below:
Related course:
PyQt5 grid layout example:
The example below creates the grid:
|
Explanation
We import the gridlayout and others with:
|
In the method createGridLayout() we create the grid with a title and set the size.
|
Widgets are added using
|
Finally we set the layout.
Several buttons are added to the grid. To add a button click action you need a pyqtslot.
If you are new to programming Python PyQt, I highly recommend this book.
Qt4 window
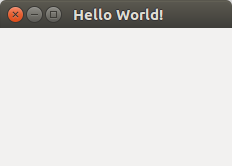
In this tutorial you will learn how to create a graphical hello world application with PyQT4.
PyQT4, it is one of Pythons options for graphical user interface (GUI) programming.
Related course:
PyQt4 window example:
This application will create a graphical window that can be minimized, maximimzed and resized it.
|
The PyQT4 module must be immported, we do that with this line:
|
We create the PyQT4 application object using QApplication():
|
We create the window (QWidget), resize, set the tittle and show it with this code:
|
Don’t forget to show the window:
|
You can download a collection of PyQt4 examples:
Download PyQT Code (Bulk Collection)
qt message box
PyQT4 offers message box functionality using several functions.
Messageboxes included in PyQT4 are: question, warning, error, information, criticial and about box.
Related course: Create GUI Apps with Python PyQt5
PyQt4 mesagebox
The code below will display a message box with two buttons:#! /usr/bin/env python |
Result:
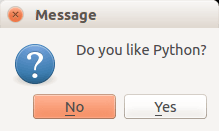
There are different types of messageboxes that PyQT4 provides.
PyQT4 Warning Box
You can display a warning box using this line of code:
QMessageBox.warning(w, "Message", "Are you sure you want to continue?") |
PyQT4 Information box
We can display an information box using QMessageBox.information()
QMessageBox.information(w, "Message", "An information messagebox @ pythonspot.com ") |
Result:
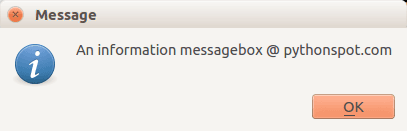
PyQT4 Critical Box
If something goes wrong in your application you may want to display an error message.
QMessageBox.critical(w, "Message", "No disk space left on device.") |
Result:
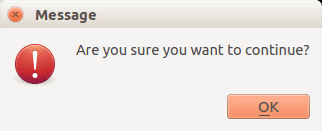
PyQT4 About box
We have shown the question box above.
QMessageBox.about(w, "About", "An example messagebox @ pythonspot.com ") |
Result:
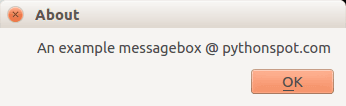
Download PyQT Code (Bulk Collection)
pyqt widgets
QT4 Table
We can show a table using the QTableWidget, part of the PyQt module. We set the title, row count, column count and add the data.
Related course:
Qt4 Table example
An example below:
from PyQt4.QtGui import * |
Result:
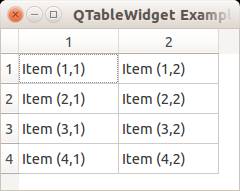
QTableWidget labels
You can set the header using the setHorizontalHeaderLabels() function. The same applies for vertical labels. A qt4 demonstration below:
from PyQt4.QtGui import * |
Result:
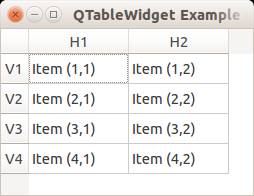
Note: These days you can use pyqt5 to create a pyqt table.
QTableWidget click events
We can detect cell clicks using this procedure, first add a function:
# on click function |
Then define the function:
def cellClick(row,col): |
The Python programming language starts counting with 0, so when you press on (1,1) you will see (0,0). Full code to detect table clicks:
from PyQt4.QtGui import * |
If you want to show the cell/row numbers in a non-programmer way use this instead:
def cellClick(row,col): |
Tooltip text
We can set tooltip (mouse over) text using the method. If you set tooltips on non-existing columns you will get an error.
from PyQt4.QtGui import * |
Result:
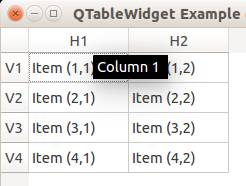
Download PyQT Code (Bulk Collection)
pyqt tabs
open file python
In this short tutorial you will learn how to create a file dialog and load its file contents. The file dialog is needed in many applications that use file access.
Related course:
File Dialog Example
To get a filename (not file data) in PyQT you can use the line:
filename = QFileDialog.getOpenFileName(w, 'Open File', '/') |
If you are on Microsoft Windows use
filename = QFileDialog.getOpenFileName(w, 'Open File', 'C:\') |
An example below (includes loading file data):
#! /usr/bin/env python |
Result (output may vary depending on your operating system):
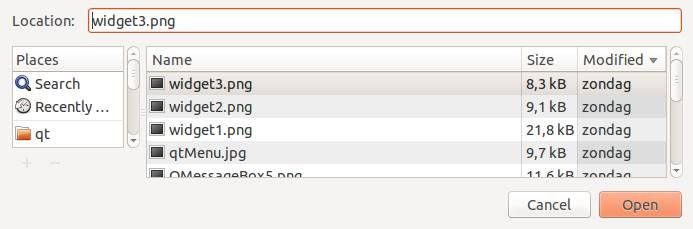